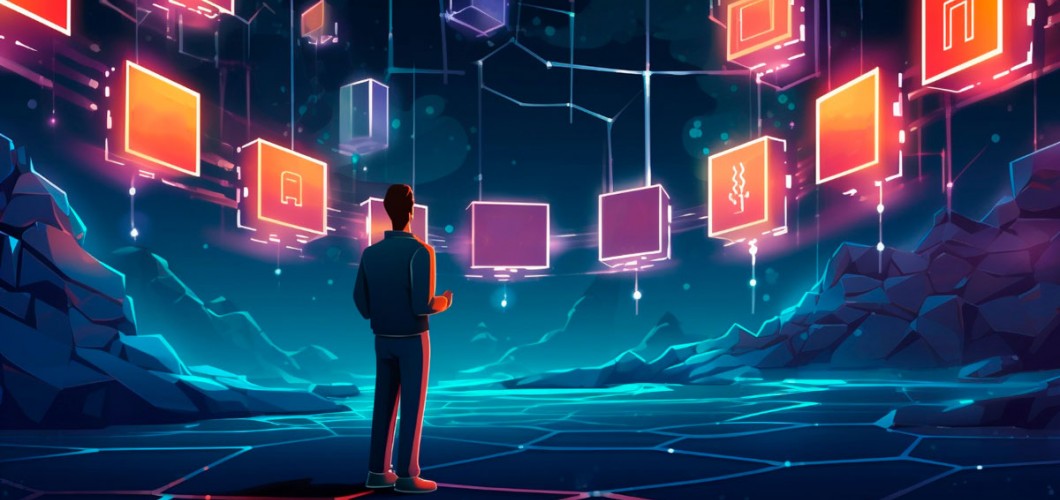
Building an API with Express.js
An API (Application Programming Interface) is an intermediary that allows different software components to interact with each other. APIs are a fundamental part of modern web and mobile applications. In this blog, we will go step-by-step to create a fast and efficient API using Express.js, a Node.js framework, explaining each step in detail and providing practical examples.
1. What is Express.js?
Express.js is a web framework for Node.js. It allows you to build APIs for web and mobile applications quickly and efficiently with its minimal and flexible design. Express provides various features to manage HTTP requests, handle routing, and add functionalities to your application using middleware.
Key advantages of Express.js:
- Simple and understandable API.
- Fast development process.
- Middleware support.
- Modular structure and extensive plugin support.
Express.js is especially useful for small to medium-sized projects, but it is also highly scalable for large and complex applications.
2. What is an API and Why is API Development Important?
An API is a tool that enables software components to communicate with each other. Nowadays, almost all applications use APIs to share data. An API provides communication between the client (frontend) and the server (backend) of an application.
Why is API development important?
- Data Sharing: Allows sharing data across different platforms.
- Independent Development: APIs allow frontend and backend to develop independently of each other.
- Efficiency: Reduces code duplication and allows for faster development.
- Scalability: Enables applications and services to scale independently.
3. Steps to Build an API with Express.js
3.1. Installing Required Tools
Before starting, make sure you have Node.js installed on your machine. Node.js is the environment that allows us to run Express.js.
- Install Node.js: Node.js Download Link
Once Node.js is installed, let’s start a new project directory.
- Create Project Directory:
mkdir express-api
cd express-api
- Initialize Node.js Project:
npm init -y
- Install Express.js:
npm install express
This command will add Express.js to our project.
3.2. Creating a Simple API
To create your first API with Express.js, follow these steps:
- Create app.js file:
// app.js
const express = require('express');
const app = express();
// Middleware to handle JSON data
app.use(express.json());
// Simple GET request
app.get('/', (req, res) => {
res.send('Hello, Express API!');
});
// Start API server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}...`);
});
The above code starts a simple Express.js API server. A GET request to the /
endpoint will return "Hello, Express API!".
3.3. HTTP Requests and CRUD Operations
APIs are typically used to perform CRUD operations (Create, Read, Update, Delete). Let’s explore how to perform these operations using Express.js.
3.3.1. Create (Add Data) - POST
To add data, we use a POST request. Here’s a simple example:
// users.json (simulating a database)
let users = [
{ id: 1, name: 'Ali', age: 25 },
{ id: 2, name: 'Ayşe', age: 30 }
];
// Create User API (POST)
app.post('/users', (req, res) => {
const { name, age } = req.body;
const newUser = { id: users.length + 1, name, age };
users.push(newUser);
res.status(201).json(newUser);
});
In this example, we add a new user by sending a POST request with name
and age
data, which will be added to the users
array.
3.3.2. Read (Fetch Data) - GET
To fetch data, we use a GET request. Here’s an example that fetches all users:
// Get all users API (GET)
app.get('/users', (req, res) => {
res.status(200).json(users);
});
This API will return the list of all users in JSON format.
3.3.3. Update (Modify Data) - PUT
To modify an existing user's data, we use a PUT request:
// Update User API (PUT)
app.put('/users/:id', (req, res) => {
const { id } = req.params;
const { name, age } = req.body;
const user = users.find(user => user.id == id);
if (user) {
user.name = name || user.name;
user.age = age || user.age;
res.status(200).json(user);
} else {
res.status(404).send('User not found');
}
});
In this example, we update a user's data based on their id
with the new name
and age
values.
3.3.4. Delete (Remove Data) - DELETE
To delete data, we use a DELETE request:
// Delete User API (DELETE)
app.delete('/users/:id', (req, res) => {
const { id } = req.params;
users = users.filter(user => user.id != id);
res.status(204).send();
});
This API removes a user from the users
array based on their id
, and returns an empty response if successful.
4. Tips for API Development with Express.js
Use Middleware: Express.js allows you to use middleware to add additional functionality to your API. For example, you can use middleware to verify database connections on each request.
Error Handling: It's important to have centralized error handling in your API. This way, you can manage all errors in one place rather than handling them separately for each request.
Database Integration: For real applications, you may need to integrate a database like MongoDB, PostgreSQL, or MySQL with your API. Express.js works seamlessly with databases, allowing you to perform CRUD operations on your data.
Security: When developing your API, it's important to focus on security. You can use JWT (JSON Web Tokens) for user authentication and authorization, ensuring that your API is secure.
5. Conclusion
Express.js is a powerful and fast framework that simplifies the API development process. With the use of RESTful APIs, HTTP requests, CRUD operations, and error management, you can quickly create efficient APIs. Additionally, Express’s flexible structure makes it scalable for larger projects.
Mastering API development is crucial for understanding the building blocks of modern web and mobile applications. With Express.js, you can efficiently and quickly develop robust APIs.
Leave a Comment