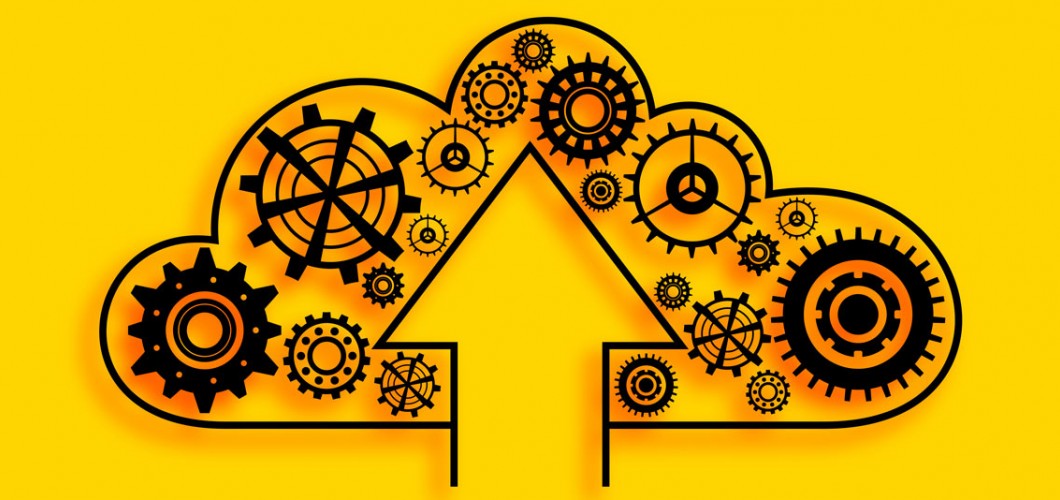
How Does the this
Keyword Work in JavaScript?
In JavaScript, the this
keyword is used to define the context (or scope) in which functions and objects operate. However, the correct understanding and usage of this
can be quite confusing, especially for beginners, as it behaves differently based on the context in which it is used. The this
keyword can refer to different objects depending on how a function or method is called, making it one of the most important features of JavaScript.
In this post, we will dive deep into what the this
keyword is, how it works, explore different usage scenarios, and highlight some potential pitfalls. We will also look at practical examples to help you understand this concept more clearly.
What is the this
Keyword?
The this
keyword in JavaScript is a special reference that changes based on the context in which a function or method is called. In simple terms, this
is used to refer to the object that the function or method is associated with. However, the value of this
depends on how the function is invoked, which can vary from one situation to another. This makes the this
keyword one of the core concepts in JavaScript.
How Does the this
Keyword Work?
The value of this
changes depending on how a function is invoked. Let’s explore how this
behaves in different scenarios, with examples.
1. this
in the Global Context
When a function is called in the global context (i.e., not as a method of an object), this
refers to the global object. In browsers, this is typically the window
object, and in Node.js, it refers to the global
object. However, in strict mode ('use strict'
), this
will be undefined
.
Example:
function globalFunction() {
console.log(this); // In the browser, it logs "window", in Node.js it logs "global"
}
globalFunction();
In this example, this
will refer to the global object (window
in browsers). However, in strict mode:
'use strict';
function globalFunction() {
console.log(this); // undefined
}
globalFunction();
In strict mode, this
will be undefined
.
2. this
Inside Object Methods
In a method of an object, this
refers to the object itself. This means that this
will point to the object that the method belongs to.
Example:
const person = {
name: 'Ahmet',
greet: function() {
console.log(`Hello, my name is ${this.name}`);
}
};
person.greet(); // Hello, my name is Ahmet
In this example, this
refers to the person
object, so this.name
accesses the name
property of the object.
3. this
in Independent Functions
When a function is called independently (i.e., not as a method of an object), this
refers to the global object (again, window
in browsers and global
in Node.js). This can lead to confusion, especially if the function is invoked in a different context.
Example:
const name = 'Ahmet';
function greet() {
console.log(`Hello, my name is ${this.name}`);
}
greet(); // Hello, my name is Ahmet (from the global object)
Here, this
refers to the global object, so it accesses the global name
variable.
4. this
in Constructor Functions
In constructor functions, this
refers to the newly created instance of the object. This is how JavaScript's object creation works in the context of constructor functions.
Example:
function Person(name, age) {
this.name = name;
this.age = age;
}
const person1 = new Person('Ahmet', 30);
console.log(person1.name); // Ahmet
In this case, this
refers to the newly created object (person1
), and properties are assigned to it.
5. this
in Arrow Functions
Arrow functions behave differently compared to regular functions. They do not have their own this
context. Instead, they inherit the this
value from the surrounding (lexical) context.
Example:
const person = {
name: 'Ahmet',
greet: function() {
const arrowFunc = () => {
console.log(`Hello, my name is ${this.name}`);
};
arrowFunc();
}
};
person.greet(); // Hello, my name is Ahmet
In this example, the arrow function inside the greet
method inherits the this
value from its parent context, which is the person
object.
6. this
with bind()
, call()
, and apply()
JavaScript provides three methods that allow you to explicitly set the value of this
: bind()
, call()
, and apply()
. These methods allow you to control the context in which a function operates, making your code more flexible.
Example: bind()
const person = {
name: 'Ahmet'
};
function greet() {
console.log(`Hello, my name is ${this.name}`);
}
const greetPerson = greet.bind(person);
greetPerson(); // Hello, my name is Ahmet
In this example, the bind()
method is used to explicitly bind the this
context to the person
object.
Example: call()
and apply()
const person1 = { name: 'Ahmet' };
const person2 = { name: 'Ali' };
function greet() {
console.log(`Hello, my name is ${this.name}`);
}
greet.call(person1); // Hello, my name is Ahmet
greet.apply(person2); // Hello, my name is Ali
Both call()
and apply()
allow you to set the value of this
manually, but call()
takes individual arguments, while apply()
takes an array of arguments.
Potential Pitfalls with this
Arrow functions behave differently: Arrow functions do not have their own
this
context, so they inherit it from their surrounding context. This can be confusing, especially when using arrow functions in event listeners or callbacks.Incorrect usage of
this
: Misusingthis
in functions that are called independently can lead to unexpected behavior. It’s important to understand how a function is being called before relying onthis
.Global context usage: When using
this
in the global context, it will reference the global object (window
in the browser,global
in Node.js). This might not be the expected behavior.
Conclusion
The this
keyword in JavaScript is a fundamental concept that defines the context in which a function or method operates. Understanding how this
works is essential for writing clean, efficient code in JavaScript. As we've seen in the examples above, this
behaves differently depending on how a function is invoked. Be mindful of how functions are called and how this
will behave in each scenario to avoid common pitfalls.
By mastering the this
keyword, you’ll be able to write more flexible and powerful JavaScript code.
Leave a Comment