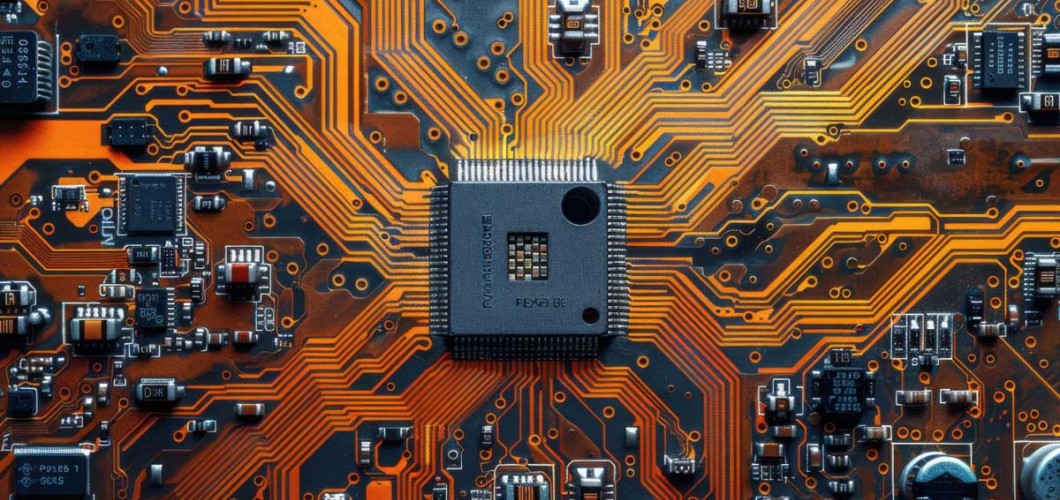
Building Desktop Applications with Electron Using JavaScript
Desktop applications are increasingly important, especially for platform independence and development speed. Electron is an open-source framework that allows you to build Windows, MacOS, and Linux desktop applications using JavaScript. Electron combines Chromium and Node.js, integrating web technologies into desktop applications. In this article, we will explain the necessary steps, core concepts, and provide examples on how to build desktop applications using Electron.
1. What is Electron?
Electron is a framework developed by GitHub that allows you to create desktop applications using JavaScript, HTML, and CSS. Electron consists of two main components:
- Chromium: The web browser engine used to render the application’s user interface.
- Node.js: A JavaScript runtime used for back-end processes.
With Electron, web developers can create desktop applications that run on multiple platforms using web technologies.
Advantages of Electron:
- Multi-Platform Support: With Electron, you can build applications that work across Windows, MacOS, and Linux with a single codebase.
- Web Technologies: Using HTML, CSS, and JavaScript to build desktop apps allows web developers to easily transition to desktop app development.
- Rich Ecosystem: Electron benefits from a vast ecosystem of modules and packages thanks to the combination of Node.js and Chromium.
2. Creating Your First Electron App
Step 1: Setting Up the Electron Project
First, make sure Node.js is installed on your machine. Then, follow these steps in your terminal or command prompt.
Create a new directory:
mkdir my-electron-app cd my-electron-app
Initialize the Node.js project:
npm init -y
Install Electron:
npm install electron --save-dev
Step 2: Creating a Simple Electron Application
Now let’s create a basic Electron app. This app will simply open a window and display a basic HTML page inside it.
Create the main file: In your project folder, create a file called
main.js
. This file will handle the main processes of the app.const { app, BrowserWindow } = require('electron'); let mainWindow; function createWindow() { mainWindow = new BrowserWindow({ width: 800, height: 600, webPreferences: { nodeIntegration: true } }); mainWindow.loadURL('https://www.example.com'); } app.whenReady().then(createWindow); app.on('window-all-closed', () => { if (process.platform !== 'darwin') { app.quit(); } });
Explanation:
BrowserWindow
is used to create a new window, andloadURL()
loads a URL in the window. In this case, we load a website.app.whenReady()
ensures thecreateWindow()
function is called when Electron is ready.app.on('window-all-closed')
ensures the application quits when all windows are closed.
Create the HTML file: Now, create an HTML file that will be loaded by the Electron app. Name this file index.html.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>My First Electron App</title> </head> <body> <h1>Welcome to My Electron App!</h1> </body> </html>
Step 3: Running Your Application
Add the following command to the scripts
section in your package.json file:
"scripts": {
"start": "electron ."
}
Now, run the app using the command:
npm start
3. Advanced Features with Electron
3.1. Customizing the Application Window
Electron offers many ways to customize the window of your desktop application. You can modify the size, add menus, and control interactions.
Example: Change the window title:
mainWindow.setTitle('My Custom Title');
3.2. Accessing the Local File System
Thanks to Node.js integration, Electron allows access to the local file system. This feature is useful for reading and writing files.
Example: Reading a file:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
3.3. Inter-Process Communication
Electron allows communication between the Main Process and Renderer Process. You can send and receive data using ipcMain
and ipcRenderer
.
Example: Sending data from the Renderer process:
// main.js
const { ipcMain } = require('electron');
ipcMain.on('send-data', (event, arg) => {
console.log(arg); // 'Hello from Renderer Process'
});
Renderer Process (index.html):
const { ipcRenderer } = require('electron');
ipcRenderer.send('send-data', 'Hello from Renderer Process');
4. Packaging and Distribution with Electron
Electron provides various tools and methods for distributing your app. You can package your Electron app using tools like electron-packager
or electron-builder
.
Example:
Packaging with
electron-packager
:npm install electron-packager --save-dev npx electron-packager . my-electron-app --platform=win32 --arch=x64
Distributing with
electron-builder
:npm install electron-builder --save-dev
5. Conclusion
Electron allows web developers to easily create cross-platform desktop applications. By using web technologies, you can develop desktop apps that run on different platforms. In this article, we explored how to create a simple Electron app, its features, and the packaging and distribution process. Now, you can start building powerful desktop applications with Electron!
Leave a Comment