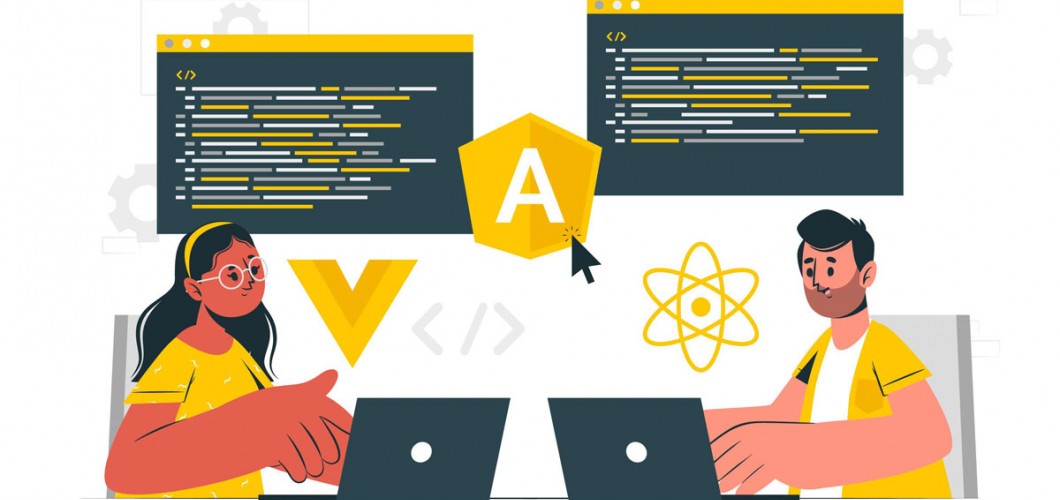
Asynchronous Programming in JavaScript: Callbacks, Promises, and Async/Await
JavaScript is a highly popular language, especially in web development. Modern web applications often rely on asynchronous operations to provide fast and dynamic user experiences. Asynchronous programming allows parts of a program to continue running without blocking the main execution thread while waiting for a task to complete. In this blog, we will explain the core concepts, methods, and examples of asynchronous programming in JavaScript: Callbacks, Promises, and Async/Await.
1. What is Asynchronous Programming?
Asynchronous programming allows parts of a program to run independently of others. For example, when you send a request to a web API, your application doesn’t freeze while waiting for a response. This is crucial for time-consuming operations (like fetching data, reading files, API calls, etc.).
The Principle of Asynchronous Operations
Asynchronous operations are used when there is a time difference between the part that starts an operation and the part that waits for the result. For example:
- The user sends a request to the database.
- The operation starts but doesn't return immediately.
- The user interface continues other operations.
- When the result is received, a callback function is triggered.
To handle asynchronous programming in JavaScript, there are three main techniques: Callbacks, Promises, and Async/Await.
2. Callbacks
A callback is a function passed as an argument to another function, and it is typically called when a certain task completes. Callbacks allow us to work with asynchronous operations. However, in more complex applications, "callback hell" can occur, which happens when multiple callbacks are nested inside one another, making the code harder to read and manage.
Callback Example
// Asynchronous function to fetch user data from a database
function getUserData(userId, callback) {
setTimeout(() => {
const userData = { id: userId, name: 'Ali' };
callback(userData); // Callback function is called here
}, 2000);
}
// Fetching user data and using a callback function
getUserData(1, function(data) {
console.log(data); // { id: 1, name: 'Ali' }
});
In this example, the getUserData
function simulates a task that takes 2 seconds. Once the operation is complete, the callback
function is invoked and the data is shown to the user.
Callback Hell
getUserData(1, function(data1) {
getUserData(2, function(data2) {
getUserData(3, function(data3) {
console.log(data1, data2, data3);
});
});
});
In this example, multiple callbacks are nested within each other, which makes the code complex and harder to maintain. This is where Promises and Async/Await come in to help.
3. Promises
Promises were introduced as an alternative to callbacks. A promise represents an asynchronous operation that will eventually complete. A promise can either be successfully completed (resolved) or it can fail (rejected). Promises provide a cleaner structure and help avoid the callback hell.
Promise Structure
let myPromise = new Promise(function(resolve, reject) {
let success = true;
if (success) {
resolve("Operation successful!");
} else {
reject("Operation failed.");
}
});
A Promise can be in one of three states:
- Pending: The operation is still in progress.
- Fulfilled: The operation has completed successfully.
- Rejected: The operation failed.
Using Promises
function getUserData(userId) {
return new Promise((resolve, reject) => {
setTimeout(() => {
const userData = { id: userId, name: 'Ali' };
resolve(userData); // Operation is successful
}, 2000);
});
}
getUserData(1)
.then((data) => {
console.log(data); // { id: 1, name: 'Ali' }
})
.catch((error) => {
console.log(error); // If there's an error, it's handled here
});
In this example, the getUserData
function returns a Promise. When the operation completes successfully, the .then()
block is executed. If the operation fails, the .catch()
block handles the error. This makes the code more readable and easier to manage.
Promise Chaining
With promises, you can chain a series of asynchronous operations. Each .then()
block handles the result of the previous Promise.
getUserData(1)
.then((data) => {
console.log(data); // { id: 1, name: 'Ali' }
return getUserData(2); // Returning a new Promise
})
.then((data) => {
console.log(data); // { id: 2, name: 'Ayşe' }
})
.catch((error) => {
console.log(error);
});
4. Async/Await
Async/Await is a feature in JavaScript that makes asynchronous code more readable. An async
function always returns a Promise, and await
is used to pause the execution of an async
function until a Promise is resolved.
Using Async/Await
async function getUserData(userId) {
return new Promise((resolve, reject) => {
setTimeout(() => {
const userData = { id: userId, name: 'Ali' };
resolve(userData);
}, 2000);
});
}
async function displayUserData() {
try {
const data = await getUserData(1); // Waits for the Promise to resolve
console.log(data); // { id: 1, name: 'Ali' }
} catch (error) {
console.log(error); // If there's an error
}
}
displayUserData();
In the example above, the getUserData
function returns a Promise, and the displayUserData
function is defined as async
. The await
keyword waits for the Promise to resolve, which makes the code more readable.
Async/Await with Promise Chaining
With Async/Await, Promise chaining can be written much more cleanly.
async function displayUserData() {
try {
const user1 = await getUserData(1);
console.log(user1); // { id: 1, name: 'Ali' }
const user2 = await getUserData(2);
console.log(user2); // { id: 2, name: 'Ayşe' }
} catch (error) {
console.log(error);
}
}
displayUserData();
Async/Await makes asynchronous code look more like synchronous code, which reduces complexity.
5. When to Use Which?
- Callback: Useful for small, simple operations. However, in more complex scenarios, it can lead to callback hell.
- Promises: Can be used as an alternative to callbacks. They improve code readability and simplify error handling.
- Async/Await: Provides a more readable and synchronous-like way to handle Promises. It is recommended for modern JavaScript projects.
Conclusion
Asynchronous programming in JavaScript is a fundamental concept for building dynamic and responsive applications. Callbacks, Promises, and Async/Await each provide different advantages in handling asynchronous operations. Callbacks are useful for basic tasks but can become difficult to manage in complex applications. Promises and Async/Await solve many of these issues, with Async/Await providing the cleanest and most readable way to handle asynchronous operations.
By understanding and correctly using all three techniques, you can work with asynchronous tasks more efficiently and build more robust applications.
Leave a Comment