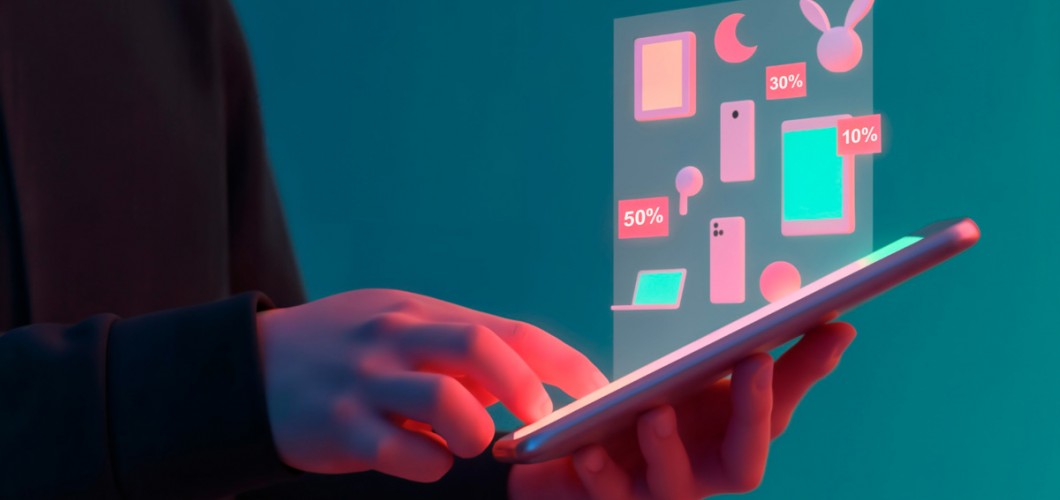
Developing Progressive Web Apps (PWA) with JavaScript
Progressive Web Apps (PWAs) are web applications developed with technology that combines the advantages of traditional web apps and the features of mobile apps. PWAs offer benefits like offline functionality, home screen installation, and push notifications. In this post, you will learn what a PWA is, how to develop one, its features, and all the information you need to build a PWA application step by step.
1. What is a PWA?
A Progressive Web App (PWA) is a web application developed using web technologies, but with integrated features of mobile apps, providing a richer, faster, and user-friendly experience. PWAs offer advantages like offline functionality, home screen installation, and push notifications.
Advantages of PWAs:
- Fast Loading: PWAs offer speed through caching and asynchronous data fetching techniques.
- Offline Functionality: PWAs allow offline usage by caching content for later use.
- Add to Home Screen: Users can add PWAs to their mobile devices’ home screen.
- Push Notifications: PWAs can send notifications to users with their permission.
2. Key Components of a PWA
To build a PWA, there are a few key components that are essential:
2.1. Service Worker
The Service Worker is a JavaScript file that runs in the background of PWAs and can interact with web pages. With Service Worker, the application can work offline, cache data, and perform background synchronization.
2.2. Web App Manifest
The Web App Manifest is a JSON file that allows PWAs to be added to the home screen and customizes the appearance of the app. This file defines the app's name, icon, start URL, and theme color.
Example Manifest File:
{
"name": "My Progressive App",
"short_name": "PWA App",
"description": "A progressive web application",
"start_url": "/",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"icons": [
{
"src": "images/icons/app-icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
}
]
}
2.3. HTTPS
PWAs must be served over HTTPS for security reasons. This is required for Service Worker and Web App Manifest to function correctly.
3. Getting Started with Developing a PWA
Let’s now build a simple PWA application, following step-by-step instructions. The steps can be applied to any real-world project.
3.1. Project Setup
First, create a directory and initialize a basic HTML app inside it.
mkdir my-pwa-app
cd my-pwa-app
touch index.html
index.html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My PWA App</title>
<link rel="manifest" href="/manifest.json">
</head>
<body>
<h1>Welcome to My PWA App!</h1>
<p>This is a simple Progressive Web App.</p>
<script src="app.js"></script>
</body>
</html>
3.2. Writing the Service Worker
Create a Service Worker file and add basic functionality:
touch service-worker.js
service-worker.js:
const CACHE_NAME = 'my-pwa-cache-v1';
const urlsToCache = [
'/',
'/index.html',
'/styles.css',
'/app.js',
];
self.addEventListener('install', (event) => {
event.waitUntil(
caches.open(CACHE_NAME)
.then((cache) => {
return cache.addAll(urlsToCache);
})
);
});
self.addEventListener('fetch', (event) => {
event.respondWith(
caches.match(event.request)
.then((response) => {
return response || fetch(event.request);
})
);
});
3.3. Registering and Activating the Service Worker
Now, register the Service Worker in your app’s JavaScript:
app.js:
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/service-worker.js')
.then((registration) => {
console.log('Service Worker registered with scope:', registration.scope);
})
.catch((error) => {
console.log('Service Worker registration failed:', error);
});
}
3.4. Web App Manifest
Create the Web App Manifest file and link it in your HTML file.
manifest.json:
{
"name": "My PWA App",
"short_name": "PWA App",
"description": "A simple progressive web application",
"start_url": "/",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"icons": [
{
"src": "images/icons/app-icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
}
]
}
4. Testing the PWA
To test your PWA:
- Open Chrome Developer Tools.
- Go to the
Application
tab and verify that your Service Worker and Manifest files are working correctly. - To test offline functionality, enable "Offline" mode in the Network tab.
5. Conclusion
Progressive Web Apps (PWAs) are a powerful way to enhance web applications by offering a native-like experience. By using JavaScript and web technologies, you can create modern apps that work across platforms. In this post, we covered the key components of a PWA, how to build one, and step-by-step instructions for creating your first PWA.
PWAs are a great approach for modern web development and can significantly improve user experience.
Leave a Comment