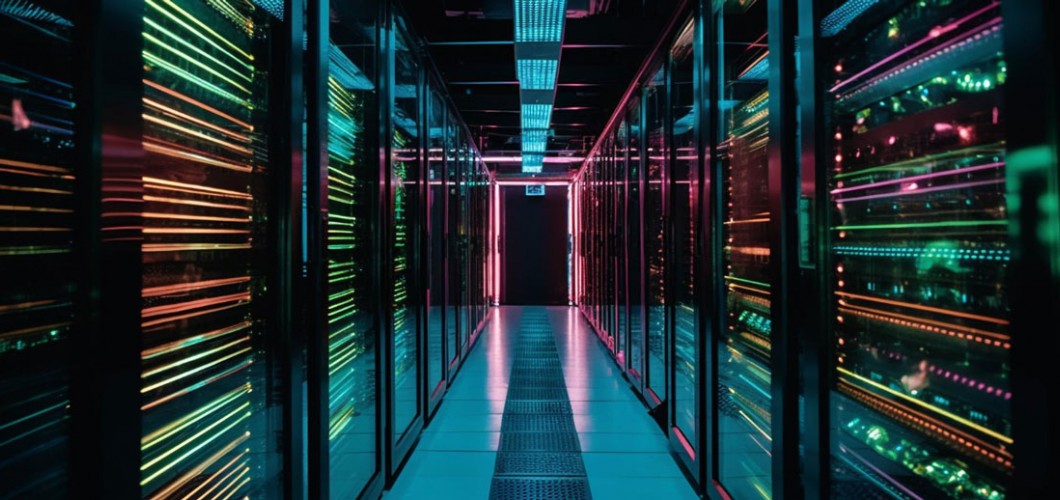
Developing SPA (Single Page Application) with Angular
A Single Page Application (SPA) is a type of web application where the entire app runs on a single page, and user interactions only modify parts of that page. In traditional multi-page applications, a new request is made to the server for every new page load, whereas in SPAs, only specific areas are updated, making the app faster and more fluid. Angular is a powerful and popular framework for building SPAs.
In this blog, we will walk you through how to develop an SPA using Angular, explaining each step with examples and visuals. We will cover everything from the basics to more advanced SPA development techniques with Angular.
1. Introduction to Angular
Angular is an open-source web framework developed by Google. It's based on TypeScript and is used for building web applications. Angular offers built-in features such as component-based architecture, routing, form management, and data binding.
SPAs are typically developed more efficiently using frameworks like Angular, as it facilitates development with efficient data binding and component-based architecture.
2. What is an SPA and Why Use It?
A SPA is a web application where the user interacts with the application without reloading the page. All content is dynamically updated by JavaScript. SPA's enhance the user experience and improve performance.
Advantages of SPAs:
- Faster Loading: Since only the necessary content is updated, the page loads faster.
- Better User Experience: User interactions happen without page reloads, providing a more seamless experience.
- Less Server Load: Instead of reloading the entire page, only the required data is fetched, reducing server load.
3. Getting Started with Angular for SPA Development
3.1. Creating an Angular Project
The first step is to create a project using Angular CLI. Angular CLI is a tool that helps you quickly set up Angular projects.
3.1.1. Installing Angular CLI
To install Angular CLI globally, run the following command in your terminal or command prompt:
npm install -g @angular/cli
3.1.2. Creating a New Angular Project
To create a new Angular project, use this command:
ng new spa-angular-app
Once the project is created, you can run your Angular application using:
cd spa-angular-app
ng serve
This will start your Angular app, which will be accessible at http://localhost:4200
.
3.2. Angular SPA Structure
In an Angular application, all components are independent, and each component performs a specific function. Angular’s component-based architecture makes it easy to develop SPA applications as each page or functional area is treated as a component, which is only rendered when needed.
In SPA development, we’ll use Angular’s Router module for navigation between pages.
4. Routing Between Pages with Angular Router
4.1. Installing Angular Router
Angular Router manages navigation between pages in SPAs. To use Angular Router, we need to import the required module into our project.
Add the Router module to app.module.ts
:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
4.2. Configuring Routing
Once Router is enabled, we need to configure routing in the app-routing.module.ts
file. Here’s a simple routing configuration:
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { HomeComponent } from './home/home.component';
import { AboutComponent } from './about/about.component';
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
This code configures routing for two pages: HomeComponent and AboutComponent.
4.3. Navigating Between Pages
To navigate between pages, use links in your app:
<!-- app.component.html -->
<nav>
<a routerLink="/">Home</a>
<a routerLink="/about">About</a>
</nav>
<router-outlet></router-outlet>
In the above code, router-outlet
is where the content for each route will be dynamically loaded.
5. Using Components and Data Binding
Since Angular follows a component-based architecture, each page or functional area is handled by a component. In these components, data binding is used to synchronize the UI with the data behind the scenes.
5.1. Example of Data Binding
Angular offers several types of data binding. Here’s an example of two-way data binding:
<!-- app.component.html -->
<div>
<h1>{{ title }}</h1>
<input [(ngModel)]="title" />
</div>
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular SPA';
}
In this example, the title
variable is bound to the HTML input element using two-way data binding.
6. Performance and Optimization
SPAs are fast as they don’t require page reloads, but performance can become an issue in larger applications. Here are a few tips for optimizing Angular SPAs:
Lazy Loading: Load only the necessary components of your application, which can reduce initial loading time. Angular supports lazy loading to load modules only when needed.
AOT (Ahead-of-Time) Compilation: Using AOT compilation can make your app load faster as Angular compiles the components and templates beforehand.
Change Detection: Angular’s change detection mechanism checks all components whenever there is a change. You can optimize this process by using
ChangeDetectionStrategy.OnPush
.
7. Conclusion
Angular SPA development provides a smooth, fast, and user-friendly experience, especially for modern web applications. With Angular’s component-based architecture, router module, and data binding features, building SPAs becomes easier and more efficient. By applying performance optimizations, you can ensure your application runs at its best.
In this blog, you’ve learned the fundamentals of SPA development with Angular, including routing, components, and data binding. With this knowledge, you have a solid foundation to build more complex Angular projects.
Visuals:
- Angular SPA Architecture: A visual representation of Angular’s component-based architecture.
- Routing Flow Diagram: A visual explanation of how routing works between pages in an Angular SPA.
I hope this blog helps you understand how to build SPAs with Angular! If you have any questions, feel free to ask.
Leave a Comment