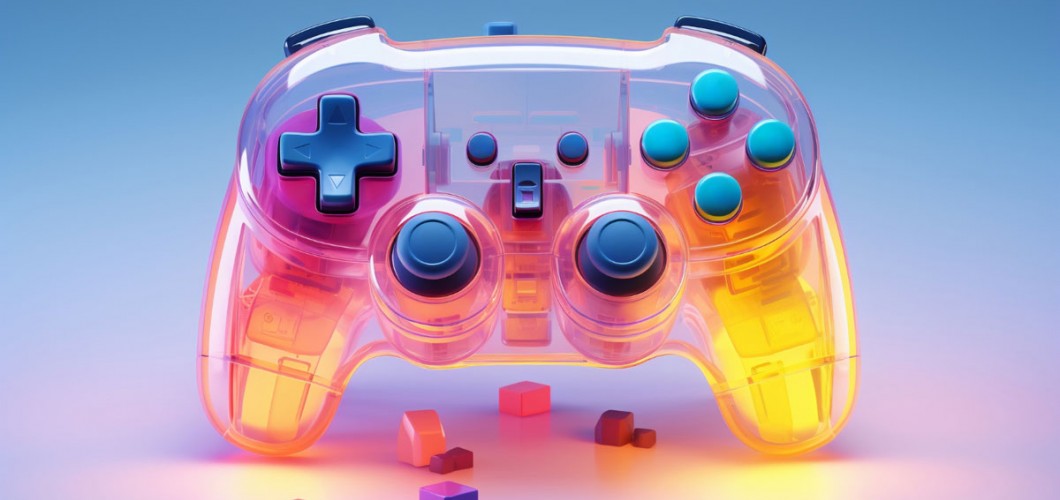
Game Development with JavaScript: Phaser Framework
Game development is a fun and educational field of software development. JavaScript is a powerful language not only for modern web applications but also for game development. In this blog post, we will explain in detail, step by step, how to develop games using Phaser Framework with JavaScript.
1. What is Phaser Framework?
Phaser is an open-source JavaScript game engine used for developing HTML5-based 2D games. It's ideal for those who want to develop games that run in web browsers and works smoothly on both mobile and desktop platforms.
1.1. Features of Phaser Framework
- Easy Setup: Easily integrable with basic JavaScript knowledge.
- High Performance: Offers high performance with WebGL and Canvas support.
- Extensive Plugin Support: Supports a wide range of modules like sound, animations, and physics engines.
- Community and Support: Active developer community and comprehensive documentation.
2. Setting Up Phaser
Follow these steps to start using Phaser.
2.1. Setup with CDN
For a quick start, use the CDN method:
<script src="https://cdn.jsdelivr.net/npm/phaser@3/dist/phaser.js"></script>
2.2. Setup with NPM
For Node.js-based projects, use NPM for setup:
npm install phaser
3. Developing a Simple Phaser Game
Let's develop a simple 2D game where a character moves around.
3.1. Project File Structure
- index.html
- main.js
- assets/
- player.png
- background.png
3.2. HTML File (index.html)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Game with Phaser</title>
<script src="https://cdn.jsdelivr.net/npm/phaser@3/dist/phaser.js"></script>
<script src="main.js" defer></script>
</head>
<body>
</body>
</html>
3.3. JavaScript File (main.js)
const config = {
type: Phaser.AUTO,
width: 800,
height: 600,
physics: {
default: 'arcade',
arcade: {
gravity: { y: 0 },
debug: false
}
},
scene: {
preload: preload,
create: create,
update: update
}
};
const game = new Phaser.Game(config);
let player;
function preload() {
this.load.image('background', 'assets/background.png');
this.load.image('player', 'assets/player.png');
}
function create() {
this.add.image(400, 300, 'background');
player = this.physics.add.sprite(400, 300, 'player');
player.setCollideWorldBounds(true);
}
function update() {
const cursors = this.input.keyboard.createCursorKeys();
if (cursors.left.isDown) {
player.setVelocityX(-160);
} else if (cursors.right.isDown) {
player.setVelocityX(160);
} else {
player.setVelocityX(0);
}
if (cursors.up.isDown) {
player.setVelocityY(-160);
} else if (cursors.down.isDown) {
player.setVelocityY(160);
} else {
player.setVelocityY(0);
}
}
4. Enhancing Game Mechanics
4.1. Adding Collision System
Add a collision system in the game as follows:
this.physics.add.collider(player, obstacle);
4.2. Adding a Scoring System
To implement a scoring system:
let score = 0;
let scoreText;
function create() {
scoreText = this.add.text(16, 16, 'Score: 0', { fontSize: '32px', fill: '#fff' });
}
function updateScore() {
score += 10;
scoreText.setText('Score: ' + score);
}
5. Advanced Game Features with Phaser
- Sound Effects: Enhance the game experience by adding sound files.
- Animations: Create sprite animations.
- Level System: Add different levels and challenges.
- Multiplayer Support: Create a multiplayer experience with WebSockets.
- Scoreboards: Record and display players' achievements.
- Difficulty Levels: Increase the difficulty level as the game progresses.
6. Conclusion
Phaser allows you to develop 2D games quickly and effectively with JavaScript. This guide provided you with the basics. You can enhance your experience by developing more complex projects.
Remember, the best way to learn is by doing. Start using Phaser to bring your game ideas to life!
Leave a Comment