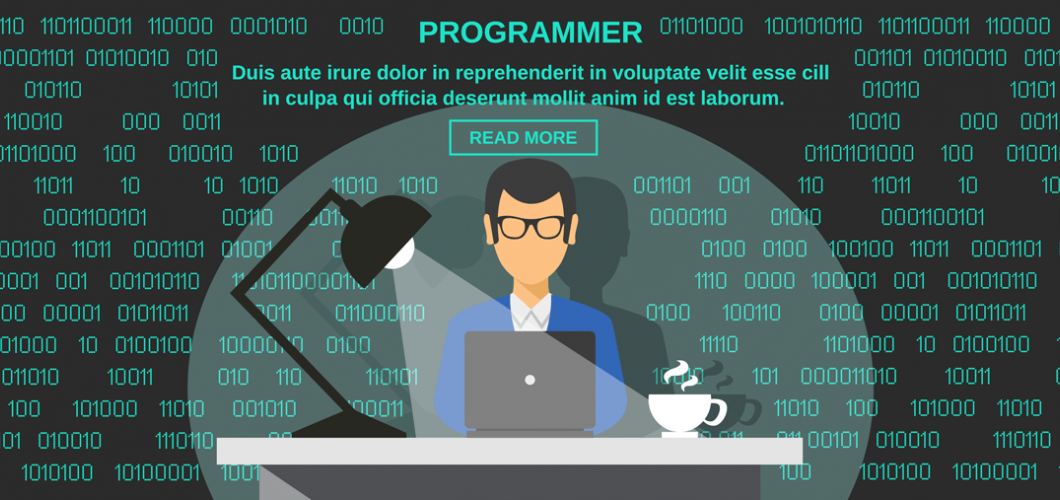
Higher-Order Functions in JavaScript
JavaScript is a flexible and powerful language that supports many advanced programming concepts. One of these concepts is higher-order functions (HOF), which represent one of the most powerful and useful features in JavaScript. In this post, we will explore what higher-order functions are, how they work, and how to use them effectively in JavaScript.
What is a Higher-Order Function?
A higher-order function is a function that can take another function as an argument or return a function as a result. In other words, higher-order functions are functions that can operate on other functions. Since JavaScript functions are first-class citizens, they can be assigned to variables, passed as arguments to other functions, and returned from functions.
Characteristics of Higher-Order Functions
- Can accept another function as an argument: A higher-order function can accept another function as an argument. This allows for more flexible and reusable code.
- Can return a function: A higher-order function can return a function, which allows for dynamic function creation.
- Can perform operations between functions: A function can perform operations between other functions, allowing for more complex behavior.
How Do Higher-Order Functions Work?
To understand how higher-order functions work, let's take a look at the following example:
function greeting(name) {
return `Hello, ${name}!`;
}
function printMessage(func) {
console.log(func('John'));
}
printMessage(greeting); // "Hello, John!"
In this example, the printMessage
function takes another function, greeting
, as an argument, calls it, and logs the output. This is an example of how a higher-order function works.
Another example is when a function returns another function:
function multiplyBy(multiplier) {
return function(number) {
return number * multiplier;
};
}
const multiplyBy2 = multiplyBy(2);
console.log(multiplyBy2(5)); // 10
In this case, the multiplyBy
function is a higher-order function because it returns another function that performs multiplication.
Use Cases of Higher-Order Functions
1. Array Operations
JavaScript provides powerful array methods that use higher-order functions to operate on arrays. For example, map
, filter
, and reduce
methods use higher-order functions to process arrays.
a. map()
The map()
method creates a new array by applying a given function to every element in an array. The function is applied to each element as an argument. Here's an example:
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map(function(number) {
return number * 2;
});
console.log(doubledNumbers); // [2, 4, 6, 8, 10]
In this example, the map()
method is a higher-order function that doubles each number in the array.
b. filter()
The filter()
method creates a new array containing only the elements that satisfy a condition. The function passed to filter()
is a higher-order function that filters the elements based on a condition:
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(function(number) {
return number % 2 === 0;
});
console.log(evenNumbers); // [2, 4]
In this example, the filter()
method filters the array and returns only even numbers.
c. reduce()
The reduce()
method reduces an array to a single value by applying a function to each element. Here's an example using reduce()
with a higher-order function:
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce(function(accumulator, currentValue) {
return accumulator + currentValue;
}, 0);
console.log(sum); // 15
In this example, the reduce()
method uses a higher-order function to sum the elements of the array.
2. Callback Functions
In JavaScript, callback functions are commonly used for asynchronous operations, and these functions are typically higher-order functions. For example, the setTimeout
function takes a callback function:
function greet(name, callback) {
console.log(`Hello, ${name}`);
callback();
}
greet('John', function() {
console.log('Good Morning!');
});
In this example, the greet
function takes a callback function as an argument and calls it after performing an action.
3. Functional Programming Techniques
JavaScript supports functional programming, and higher-order functions are at the core of functional programming techniques. Higher-order functions allow us to write more abstract and generalized functions. For example, you can create a chain of operations:
function add(x) {
return function(y) {
return x + y;
};
}
const add5 = add(5);
console.log(add5(10)); // 15
In this example, the add
function is a higher-order function that returns a function, creating a chain of operations.
Higher-Order Functions and Performance
While higher-order functions are powerful, there are some performance considerations to keep in mind. Since each function call involves an operation, using too many functions can sometimes lead to small performance overhead. However, JavaScript's V8 engine optimizes functions very efficiently, so in most cases, using higher-order functions does not negatively impact performance. In fact, higher-order functions generally make the code more readable and accelerate development time.
Common Mistakes with Higher-Order Functions
- Excessive Global Variable Access: While higher-order functions are powerful, improperly referencing too many global variables in them can lead to performance issues and unintended side effects.
- Memory Consumption: Since higher-order functions often return other functions, they can hold references to variables, leading to unnecessary memory consumption if not properly managed.
Conclusion
Higher-Order Functions (HOFs) are one of the most powerful features of JavaScript. They allow us to work with functions more flexibly and abstractly. Higher-order functions enable us to pass functions as arguments, return functions, and perform operations between functions. They are widely used in array operations, callback functions, and functional programming techniques, making the code more modular, reusable, and expressive.
By mastering higher-order functions, you can make your code more flexible, maintainable, and efficient. Now that you understand how higher-order functions work, you can leverage their power to write cleaner and more effective code in JavaScript.
Leave a Comment