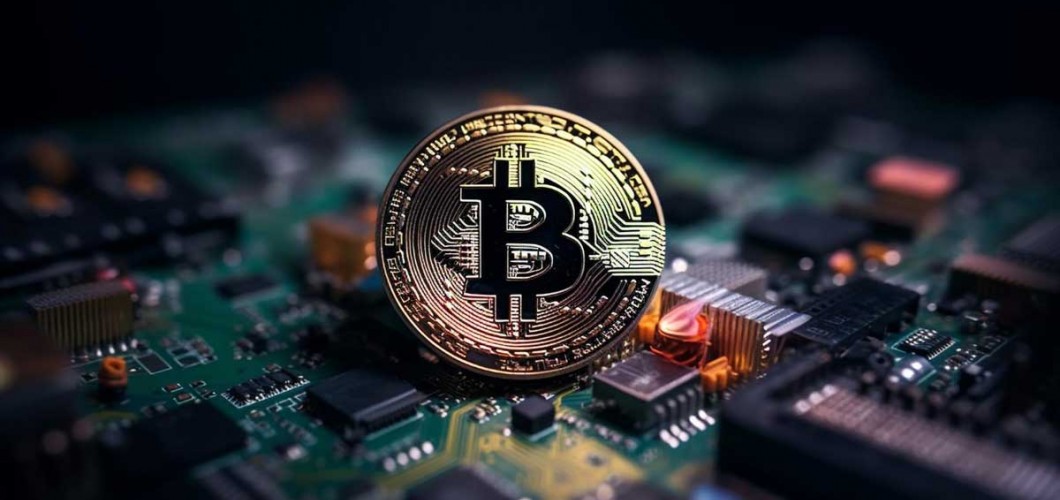
JavaScript and Blockchain: Writing Smart Contracts
Blockchain technology has rapidly gained popularity in recent years. With advanced features such as smart contracts, blockchain has significantly expanded its potential, especially in financial applications. While JavaScript is typically used for web development, it has also found its place in blockchain application development. In this article, we will explore how to write smart contracts using JavaScript in detail.
1. What is Blockchain?
Blockchain is a data structure where data is stored in connected "blocks". These blocks store transaction records, and each block is linked to the previous block, forming a chain. Since blockchain has a decentralized structure, it ensures secure and transparent data storage.
Blockchain is used beyond financial systems, including in supply chain management, healthcare, and smart contracts. Smart contracts are programs that automatically execute on the blockchain when certain conditions are met.
2. What are Smart Contracts?
Smart contracts are digital programs that implement conditional agreements on a blockchain network. These contracts are used to increase trust between parties and eliminate the need for intermediaries. Smart contracts define the terms of an agreement in software code, and once the specified conditions are met, they execute automatically.
Ethereum is one of the most popular blockchain platforms for writing smart contracts. The Ethereum network supports writing smart contracts with a language called Solidity. However, JavaScript can also be used to interact with and manage smart contracts on Ethereum.
3. JavaScript and Blockchain: Essential Tools
Although JavaScript is typically used for frontend development, it also provides powerful tools for interacting with blockchain technology. Here are the most commonly used JavaScript tools for interacting with the blockchain and managing smart contracts:
3.1. Web3.js
Web3.js is the most popular JavaScript library for interacting with the Ethereum blockchain. Web3.js allows you to connect to the Ethereum network, interact with smart contracts, send transactions, and more. It is widely used for developing decentralized applications (dApps) that run on Ethereum.
Connecting to Ethereum with Web3.js
The following example demonstrates how to connect to the Ethereum network using Web3.js:
// Include the Web3.js library
const Web3 = require('web3');
// Create a Web3 instance to connect to the Ethereum network
const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID');
// Ethereum wallet address
const address = '0xYourEthereumAddress';
// Get wallet balance
web3.eth.getBalance(address).then(balance => {
console.log('Wallet Balance:', web3.utils.fromWei(balance, 'ether'), 'ETH');
});
This code connects to the Ethereum main network using Web3.js and retrieves the balance of the specified address.
3.2. ethers.js
ethers.js is another popular JavaScript library used as an alternative to Web3.js. It is a smaller and faster library, specifically designed for interacting with the Ethereum blockchain. It also provides tools for interacting with smart contracts.
Making a Smart Contract Call with ethers.js
Here is an example of how to interact with a smart contract on Ethereum using ethers.js:
const { ethers } = require('ethers');
// Connect to the Ethereum network
const provider = new ethers.JsonRpcProvider('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID');
// Smart contract address and ABI (Application Binary Interface)
const contractAddress = '0xYourSmartContractAddress';
const abi = [
// ABI of the smart contract
"function getBalance(address _owner) public view returns (uint256)"
];
// Create a smart contract instance
const contract = new ethers.Contract(contractAddress, abi, provider);
// Fetch data from the smart contract
async function getBalance() {
const balance = await contract.getBalance('0xYourEthereumAddress');
console.log('Your balance in the smart contract:', ethers.utils.formatEther(balance));
}
getBalance();
This code demonstrates how to fetch data from a smart contract using ethers.js.
4. Writing Smart Contracts: Solidity
Smart contracts are typically written in a language called Solidity. Solidity is a language used to write smart contracts that run on the Ethereum blockchain. It allows you to define the terms of a contract in code.
4.1. Simple Smart Contract with Solidity
Below is an example of a simple smart contract written in Solidity. This contract is used to store a user's balance:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 public storedData;
// Function to set a value
function set(uint256 x) public {
storedData = x;
}
// Function to get the value
function get() public view returns (uint256) {
return storedData;
}
}
This contract allows you to store and retrieve a value using the set
and get
functions.
5. Interacting with Smart Contracts via JavaScript
JavaScript, through libraries like Web3.js and ethers.js, allows interaction with Solidity-based smart contracts. These libraries provide the necessary tools to connect to Ethereum, send transactions, and interact with smart contracts.
Sending Data to a Smart Contract with Web3.js
The following example demonstrates how to send data to an Ethereum smart contract using Web3.js:
const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID');
const contractAddress = '0xYourSmartContractAddress';
const abi = [
// ABI of the smart contract
"function set(uint256 x) public"
];
// Create the contract instance
const contract = new web3.eth.Contract(abi, contractAddress);
// Wallet address and sending transaction
const account = '0xYourEthereumAddress';
const privateKey = '0xYourPrivateKey';
web3.eth.accounts.signTransaction({
to: contractAddress,
data: contract.methods.set(100).encodeABI(),
gas: 2000000
}, privateKey).then(signedTx => {
web3.eth.sendSignedTransaction(signedTx.rawTransaction);
});
This code sends data to the smart contract and executes the set
function to add a specific value.
6. Conclusion
JavaScript is a powerful language that can be effectively integrated with blockchain technology. Using libraries like Web3.js and ethers.js, JavaScript enables interaction with smart contracts and facilitates blockchain application development. Smart contracts provide a secure and automatic way to execute transactions on the blockchain and serve as the foundation of decentralized applications (dApps).
Combining blockchain and JavaScript gives developers great flexibility in building blockchain-based applications for both the frontend and backend.
1 Comment(s)
Başarılı
Leave a Comment