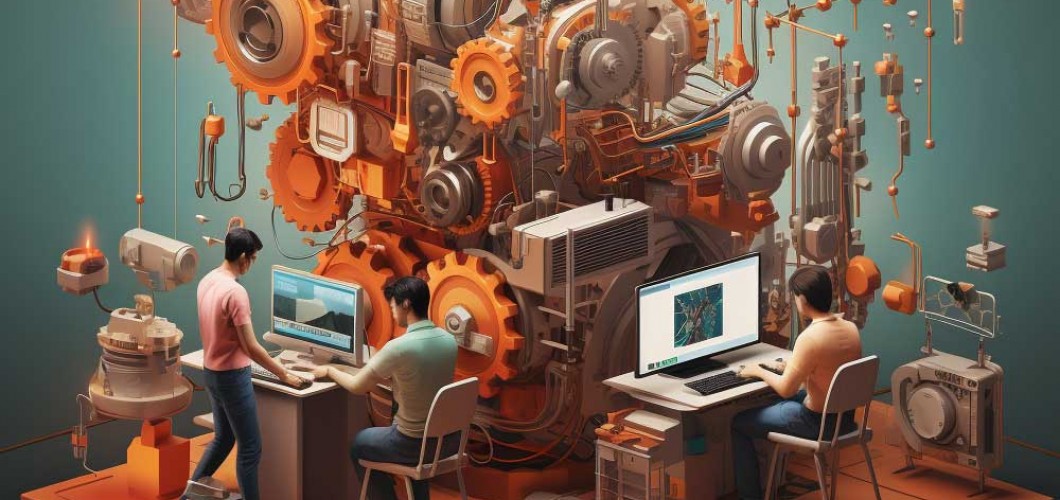
JavaScript and DOM (Document Object Model) Manipulation
One of the core components of web applications is JavaScript, which interacts with page content and provides users with a dynamic experience. JavaScript allows you to manage user interactions on web pages, change content dynamically, add new content, and interact with page elements. The key structure that JavaScript interacts with is the DOM (Document Object Model).
In this article, we will provide a comprehensive explanation of JavaScript and DOM manipulation, along with examples to offer a deeper understanding of the topic.
1. What is DOM (Document Object Model)?
The DOM is an object model used by browsers to represent HTML or XML documents. When a web page is loaded, each element on the page is turned into an object, and through these objects, the content of the page can be manipulated with JavaScript. The DOM represents the page structure in a tree-like manner, where each HTML element is represented as a "node."
For example, the following HTML document might look like this:
<html>
<head>
<title>DOM Manipulation</title>
</head>
<body>
<div id="container">
<h1>Hello World!</h1>
<p>This is an example.</p>
</div>
</body>
</html>
This HTML structure is represented in the DOM like this:
Document
└─ html
├─ head
│ └─ title ("DOM Manipulation")
└─ body
└─ div (id: "container")
├─ h1 ("Hello World!")
└─ p ("This is an example.")
2. Accessing and Selecting Elements in DOM
To access DOM elements with JavaScript, there are various methods available. Some of the most commonly used ones are:
2.1 getElementById
This method selects the element with the specified id
.
let element = document.getElementById('container');
console.log(element); // <div id="container">...</div>
2.2 getElementsByClassName
This method returns all elements with the specified class
name as a collection.
let elements = document.getElementsByClassName('example-class');
console.log(elements); // HTMLCollection
2.3 getElementsByTagName
This method selects all elements with a specified tag name.
let paragraphs = document.getElementsByTagName('p');
console.log(paragraphs); // HTMLCollection
2.4 querySelector
querySelector
returns the first element that matches the given CSS selector.
let header = document.querySelector('h1');
console.log(header); // <h1>Hello World!</h1>
2.5 querySelectorAll
The querySelectorAll
method returns all elements that match the given CSS selector.
let allParagraphs = document.querySelectorAll('p');
console.log(allParagraphs); // NodeList
3. DOM Manipulation
With JavaScript, various manipulations can be made on the DOM. These operations include adding elements, deleting elements, changing styles, updating text, and more.
3.1 Changing Text
To change the text inside an element, you can use the textContent
or innerHTML
properties.
let h1Element = document.querySelector('h1');
h1Element.textContent = 'New Title';
3.2 Adding Elements
To add new elements to the DOM, you can use the createElement
and appendChild
methods.
let newParagraph = document.createElement('p');
newParagraph.textContent = 'This is a new paragraph.';
document.body.appendChild(newParagraph);
3.3 Removing Elements
To remove an element from the DOM, you can use the removeChild
or remove
method.
let paragraphToRemove = document.querySelector('p');
paragraphToRemove.remove();
3.4 Adding/Removing Classes
To add or remove classes from an element, the classList
property is used. Methods like add
, remove
, and toggle
can be used to manage classes.
let divElement = document.getElementById('container');
divElement.classList.add('new-class');
divElement.classList.remove('old-class');
divElement.classList.toggle('active');
3.5 Changing Styles
To change the style of an element, you can use the style
property.
let divElement = document.querySelector('div');
divElement.style.backgroundColor = 'lightblue';
divElement.style.padding = '20px';
3.6 Event Handling
You can add event listeners to DOM elements in JavaScript. These events are triggered by user interactions. For example, to handle a click event on a button:
let button = document.querySelector('button');
button.addEventListener('click', function() {
alert('Button clicked!');
});
4. Interactive Web Pages with DOM Manipulation
JavaScript and DOM manipulation are powerful tools for creating interactive and dynamic web pages. For example, let's consider a form. When a user fills out the form, the page can provide instant feedback.
Example: Dynamically Adding Form Fields
When a user wants to add a new form field, JavaScript can dynamically update the form.
<form id="myForm">
<input type="text" name="name" placeholder="Enter your name">
<button type="button" id="addFieldBtn">Add New Field</button>
</form>
<script>
document.getElementById('addFieldBtn').addEventListener('click', function() {
let newField = document.createElement('input');
newField.type = 'text';
newField.placeholder = 'New Field';
document.getElementById('myForm').appendChild(newField);
});
</script>
In this example, when the "Add New Field" button is clicked, a new text input field is added below the form.
5. Performance and Best Practices
While DOM manipulation is powerful, it should be used with care. Improper use of DOM manipulation can negatively impact page performance. Some recommendations include:
- Avoid Excessive Reflows: Every DOM manipulation can lead to reflowing the page. Instead, try to batch DOM updates and update the page in one go.
- Use Document Fragment: If you need to add multiple elements, use
DocumentFragment
to temporarily hold the changes, then add them all at once to the DOM. - Delegated Event Handling: If you need to attach event handlers to dynamically added elements, consider delegating the events to a parent element.
Conclusion
JavaScript and DOM manipulation are crucial tools for web developers. They allow dynamic changes to page content, manage user interactions, add animations, and more. JavaScript offers powerful methods for efficiently handling DOM operations.
In this article, we covered the basics of DOM manipulation, from element selection to different methods of accessing and modifying the DOM. Understanding these techniques will help you build interactive and dynamic web pages.
Leave a Comment