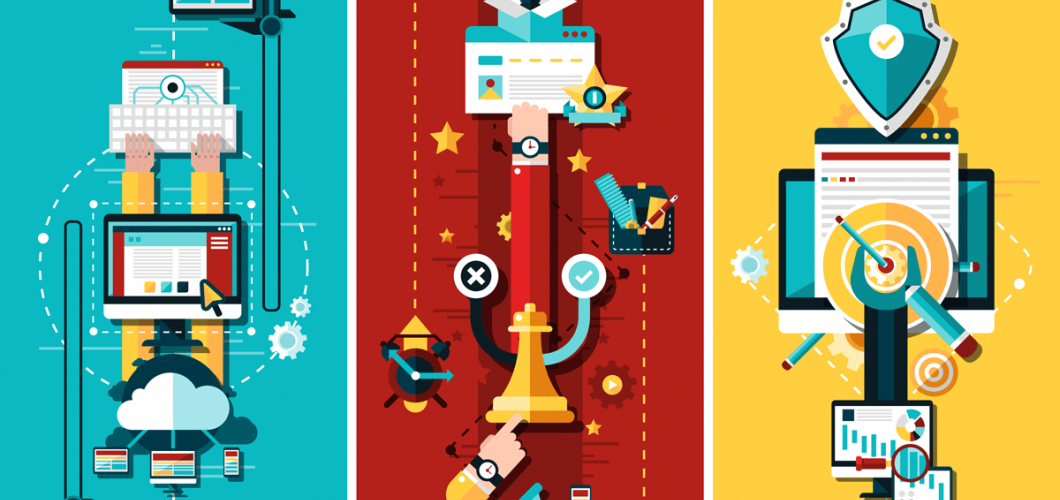
Closure Concept in JavaScript and Its Use Cases
JavaScript is a flexible and powerful language that contains many advanced programming concepts. One of these concepts is closure. Closure is one of the most powerful and confusing features in JavaScript, but when understood correctly, it can offer significant advantages. In this post, we will explore what closure is, how it works, and where it can be used effectively.
What is Closure?
In JavaScript, a closure is the ability for a function to access variables from its outer scope, even after the outer function has finished executing. In simple terms, a closure allows a function to remember and access its lexical environment even when it's executed outside of that environment.
Closure is closely related to JavaScript's lexical scoping feature. Lexical scoping determines which variables are accessible by a function based on where the function is defined. This is one of the foundational features of JavaScript's functional programming capabilities.
How Closure Works
To understand how closure works, let's look at the following example:
function outer() {
let outerVariable = 'Outer Variable';
function inner() {
console.log(outerVariable);
}
return inner;
}
const closureExample = outer();
closureExample(); // "Outer Variable"
In this example, the outer
function returns the inner
function. The inner
function can still access the outerVariable
, which is defined in the outer function, even though the outer
function has finished executing. This is a fundamental example of a closure.
Closures in JavaScript are created when a function operates in a "closure context" — meaning the function can access variables from its lexical scope even after the outer function has finished executing.
Why is Closure Important?
Closures are extremely useful in certain scenarios. Here are some key use cases:
- Data Privacy: Closure helps keep certain variables private, allowing them to be accessed only within the function.
- State Management: Closures can be used to store and update state across function calls.
- Callback Functions: In asynchronous programming, closures are useful to access external variables in callback functions.
Use Cases of Closure
1. Data Privacy and Encapsulation
Closures are particularly useful for ensuring data privacy. You can hide certain variables from the outside world while still making them accessible within the function. This is a powerful technique for encapsulating data.
function createCounter() {
let count = 0; // `count` is only accessible within the `increment` function
return {
increment: function() {
count++;
console.log(count);
},
decrement: function() {
count--;
console.log(count);
},
getCount: function() {
return count;
}
};
}
const counter = createCounter();
counter.increment(); // 1
counter.increment(); // 2
counter.decrement(); // 1
console.log(counter.getCount()); // 1
In this example, the count
variable is not accessible directly from the outside, but it can be modified or read through the provided methods like increment
, decrement
, and getCount
. This ensures data privacy and encapsulation.
2. Using Closure in Callback Functions
Closure is often used in asynchronous functions, such as with setTimeout
or setInterval
, to maintain access to variables from the outer scope.
function createTimer() {
let count = 0;
setInterval(function() {
count++;
console.log(`Time: ${count} seconds`);
}, 1000);
}
createTimer(); // Logs the time every second
In this example, the closure allows the inner function to access the count
variable, even though it is part of an asynchronous setInterval
function. The closure ensures that count
is updated every second.
3. Private Methods
Closures can also be used to create private methods in objects. This means certain methods or properties are hidden from the outside world and can only be accessed through the object’s public methods.
function Car(model, year) {
let speed = 0; // `speed` is not accessible from outside the object
this.model = model;
this.year = year;
this.accelerate = function() {
speed += 10;
console.log(`Speed: ${speed} km/h`);
};
this.brake = function() {
speed -= 10;
console.log(`Speed: ${speed} km/h`);
};
}
const myCar = new Car('Toyota', 2020);
myCar.accelerate(); // Speed: 10 km/h
myCar.brake(); // Speed: 0 km/h
Here, the speed
variable is not directly accessible but can be modified through the accelerate
and brake
methods. This provides a way to keep the internal state private while allowing external interaction.
4. Event Handlers
When working with event listeners in JavaScript, closures are commonly used. This is especially true when interacting with the DOM and using external data within event handlers.
function setupButton() {
let count = 0;
const button = document.querySelector('button');
button.addEventListener('click', function() {
count++;
console.log(`Button clicked ${count} times`);
});
}
setupButton();
In this example, the closure allows the event listener to access and modify the count
variable each time the button is clicked.
Closure and Performance
While closures are powerful, they can sometimes impact memory usage. This is because closures hold references to variables from their outer scope. If not properly managed, this can lead to memory leaks. However, when used correctly, closure does not negatively impact performance and can be a very efficient tool.
Common Mistakes with Closures
- Excessive Global Variable Access: Closures can be very useful, but using them improperly (e.g., referencing too many global variables) can lead to performance problems and unwanted side effects.
- Memory Consumption: Since closures hold references to variables, large and complex closures can lead to unnecessary memory consumption.
Conclusion
The closure concept in JavaScript is a powerful feature that allows functions to access variables from their outer scope, even after the outer function has finished executing. Closures have many use cases, including data privacy, state management, callback functions, and more. When used correctly, closures can make your code more secure, efficient, and flexible. However, improper usage can lead to memory leaks and performance issues.
Closure is a core concept of JavaScript's functional programming capabilities and plays a crucial role in writing clean, modular, and maintainable code.
Leave a Comment