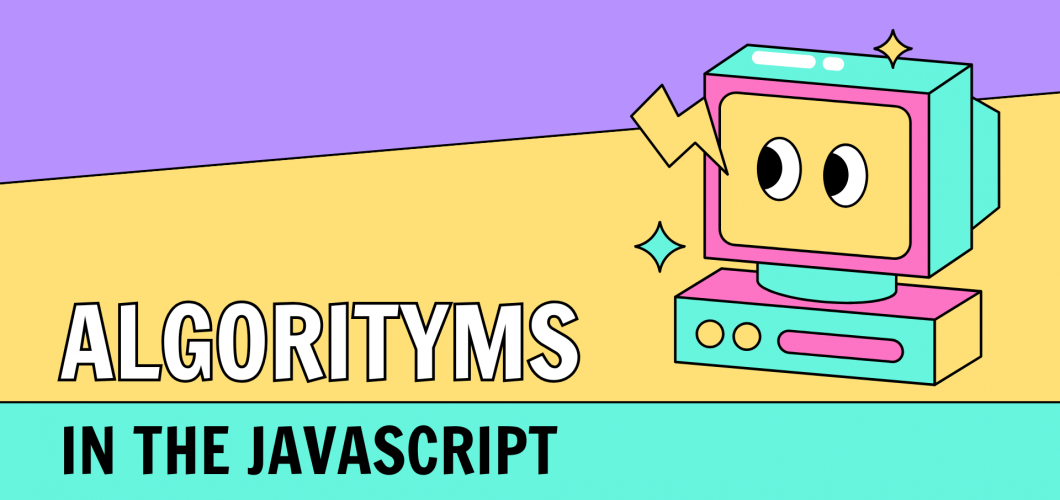
JavaScript Data Structures and Algorithms: A Comprehensive Guide
Introduction
JavaScript is not only a cornerstone of web development but also a powerful language for working with data structures and algorithms. In this article, we will explore fundamental and advanced data structures, algorithms, and their real-world applications using JavaScript.
1. Data Structures
Data structures are structural formats used to store and organize data. The most common data structures in JavaScript are:
1.1 Arrays
Arrays store multiple values in a single variable.
let numbers = [1, 2, 3, 4, 5];
console.log(numbers[0]); // 1
Array Methods:
push()
,pop()
,shift()
,unshift()
,splice()
,slice()
,map()
,filter()
,reduce()
, etc.
1.2 Linked Lists
Linked lists store elements in node structures.
class Node {
constructor(data) {
this.data = data;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
}
append(data) {
let newNode = new Node(data);
if (!this.head) {
this.head = newNode;
return;
}
let current = this.head;
while (current.next) {
current = current.next;
}
current.next = newNode;
}
}
Linked lists provide dynamic memory usage compared to arrays.
1.3 Stack
A stack operates on the LIFO (Last In, First Out) principle.
class Stack {
constructor() {
this.items = [];
}
push(element) {
this.items.push(element);
}
pop() {
return this.items.pop();
}
peek() {
return this.items[this.items.length - 1];
}
}
Real-World Applications:
- Browser "undo" functionality
- Function call stack
1.4 Queue
A queue follows the FIFO (First In, First Out) principle.
class Queue {
constructor() {
this.items = [];
}
enqueue(element) {
this.items.push(element);
}
dequeue() {
return this.items.shift();
}
}
Real-World Applications:
- Print job queues
- Data streaming systems
2. Algorithms
2.1 Sorting Algorithms
2.1.1 Selection Sort
function selectionSort(arr) {
let n = arr.length;
for (let i = 0; i < n - 1; i++) {
let minIndex = i;
for (let j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
[arr[i], arr[minIndex]] = [arr[minIndex], arr[i]];
}
return arr;
}
2.1.2 Quick Sort
function quickSort(arr) {
if (arr.length < 2) return arr;
let pivot = arr[0];
let left = arr.slice(1).filter(el => el < pivot);
let right = arr.slice(1).filter(el => el >= pivot);
return [...quickSort(left), pivot, ...quickSort(right)];
}
2.2 Searching Algorithms
2.2.1 Linear Search
function linearSearch(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) return i;
}
return -1;
}
2.2.2 Binary Search
function binarySearch(arr, target) {
let left = 0, right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
target < arr[mid] ? (right = mid - 1) : (left = mid + 1);
}
return -1;
}
Conclusion
In this article, we explored fundamental data structures and algorithms using JavaScript. Understanding these concepts is essential for optimizing development processes and writing efficient code. You can deepen your knowledge by working on practical projects.
Leave a Comment