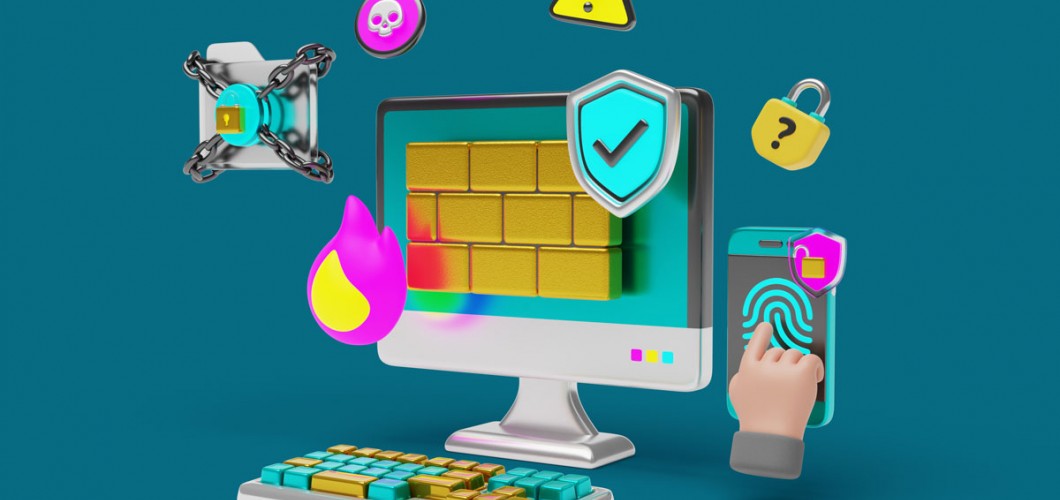
Destructuring Assignment in JavaScript: Object and Array Destructuring
JavaScript has evolved significantly in recent years, introducing many powerful features. One of the most useful and widely adopted features is Destructuring Assignment. This feature allows us to easily and clearly break apart arrays and objects into individual variables. In this article, we will explore in-depth what Destructuring Assignment is, how it works, its use cases, advantages, and examples.
What is Destructuring Assignment?
Destructuring Assignment is a feature in JavaScript that allows us to unpack elements from arrays or properties from objects into distinct variables more concisely. This technique helps in writing cleaner, more readable code when dealing with arrays and objects. Destructuring can be applied to both arrays and objects, but the syntax differs slightly for each.
Array Destructuring Assignment
With array destructuring, we can easily assign array elements to variables. This approach is especially useful when dealing with long arrays, as it makes the code more readable and shorter.
Array Destructuring Example
const array = [10, 20, 30];
// Destructuring to assign each element to a variable
const [a, b, c] = array;
console.log(a); // 10
console.log(b); // 20
console.log(c); // 30
Here, each element of the array
is assigned to a
, b
, and c
variables. This eliminates the need to access elements by their index.
Array Destructuring with Only Needed Elements
If you only want to retrieve specific elements from an array, you can just extract the needed ones.
const array = [10, 20, 30, 40, 50];
// Get only the first two elements
const [a, b] = array;
console.log(a); // 10
console.log(b); // 20
In this example, we only focus on a
and b
, skipping the rest of the elements.
Using the Rest Parameter to Get the Remaining Elements
With destructuring, we can collect the remaining elements in an array using the ...
(rest operator).
const array = [10, 20, 30, 40, 50];
// Get the first element and collect the remaining ones
const [a, b, ...rest] = array;
console.log(a); // 10
console.log(b); // 20
console.log(rest); // [30, 40, 50]
In this example, the first two elements are assigned to a
and b
, while the rest of the elements are collected into the rest
array.
Object Destructuring Assignment
With objects, destructuring allows us to extract specific properties and assign them to variables. Object destructuring is especially useful for working with large and complex objects.
Object Destructuring Example
const object = {
name: 'Ahmet',
age: 25,
city: 'Istanbul'
};
// Destructuring the object to assign its properties to variables
const { name, age, city } = object;
console.log(name); // Ahmet
console.log(age); // 25
console.log(city); // Istanbul
In this example, the properties name
, age
, and city
from the object
are directly assigned to their respective variables.
Object Destructuring with Renaming
Sometimes, you may want to assign a property to a variable with a different name. You can do this by renaming the key in the destructuring syntax.
const object = {
name: 'Ahmet',
age: 25,
city: 'Istanbul'
};
// Renaming properties during destructuring
const { name: fullName, age: years, city: location } = object;
console.log(fullName); // Ahmet
console.log(years); // 25
console.log(location); // Istanbul
Here, the name
property is renamed to fullName
, age
to years
, and city
to location
.
Using the Rest Parameter to Collect Remaining Properties
With object destructuring, you can extract certain properties and gather the remaining properties into another object using the ...
(rest operator).
const object = {
name: 'Ahmet',
age: 25,
city: 'Istanbul',
profession: 'Developer'
};
// Extract a few properties, collect the rest into a new object
const { name, age, ...others } = object;
console.log(name); // Ahmet
console.log(age); // 25
console.log(others); // { city: 'Istanbul', profession: 'Developer' }
In this example, the properties name
and age
are extracted, and the rest are collected into the others
object.
Destructuring with Functions
Destructuring can also be used with function parameters, making it easier to extract values directly from passed objects or arrays.
Destructuring with Function Parameters
function userInfo({ name, age }) {
console.log(`Name: ${name}, Age: ${age}`);
}
const user = {
name: 'Ahmet',
age: 25,
city: 'Istanbul'
};
userInfo(user); // Name: Ahmet, Age: 25
In this example, the userInfo
function directly receives an object and extracts the name
and age
properties from it.
Destructuring with Default Values
If a value in an array or object is undefined, you can set default values to prevent errors.
Array Destructuring with Default Values
const array = [10];
// Assign default value to second element
const [a, b = 20] = array;
console.log(a); // 10
console.log(b); // 20
Here, since the second element in the array is not defined, the b
variable is assigned a default value of 20
.
Object Destructuring with Default Values
const object = {
name: 'Ahmet'
};
// Assign default value to undefined property
const { name, age = 30 } = object;
console.log(name); // Ahmet
console.log(age); // 30
In this case, the age
property is not defined in the object, so the age
variable is assigned a default value of 30
.
Conclusion
Destructuring Assignment in JavaScript is a powerful feature that helps you write cleaner, more concise code. It allows for easy manipulation of arrays and objects, improving the readability and maintainability of your code. Whether you're working with large data structures or handling function parameters, destructuring can make your JavaScript code more efficient and less error-prone.
This article provided an in-depth overview of Destructuring Assignment in JavaScript. Now, you should have a deeper understanding of how to destructure arrays and objects, assign default values, and use rest parameters. Happy coding!
Leave a Comment