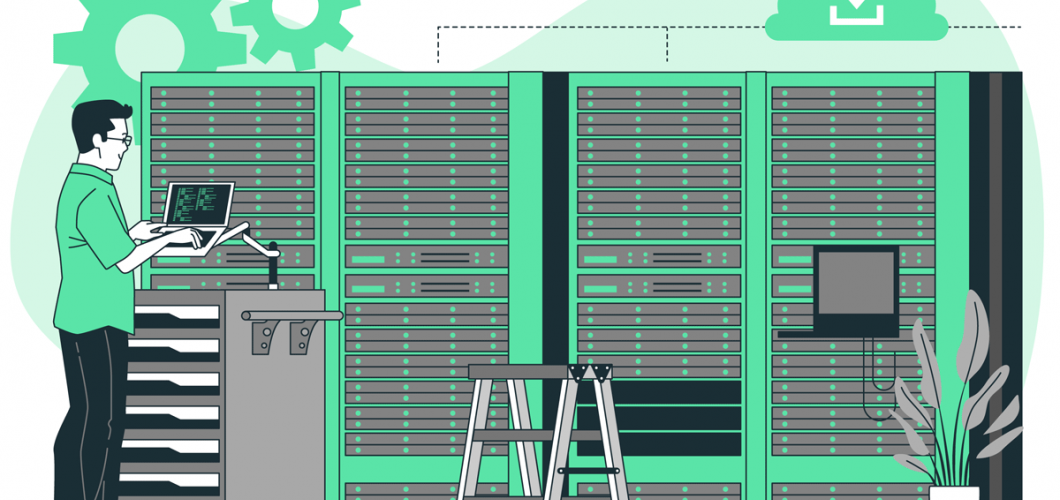
JavaScript Event Bubbling and Event Delegation
Event Bubbling and Event Delegation are important concepts when working with DOM (Document Object Model) manipulation and user interactions in JavaScript. These two concepts concern how an event propagates and how we handle it more efficiently. In this blog post, we will explain Event Bubbling and Event Delegation, discuss the differences between them, and provide examples on how to implement both techniques.
What is Event Bubbling?
Event Bubbling refers to the process where an event triggered on a target element propagates upwards to its parent elements in the DOM. In other words, the event "bubbles" from the child element upwards through its parent elements.
For example, when you click a <button>
, the event first occurs on the button itself, and then it bubbles up to the parent elements like <div>
, <form>
, and so on.
How Does Event Bubbling Work?
When an event is triggered, JavaScript handles it in two phases:
- Capture Phase: The event travels from the top-most element down to the target element.
- Bubbling Phase: The event starts from the target element and bubbles upwards to its parent elements.
Example: Event Bubbling
<!DOCTYPE html>
<html lang="tr">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Event Bubbling</title>
</head>
<body>
<div id="parent">
<button id="child">Click me!</button>
</div>
<script>
// Event listener on Parent div
document.getElementById("parent").addEventListener("click", function() {
alert("Parent clicked!");
});
// Event listener on Child button
document.getElementById("child").addEventListener("click", function() {
alert("Child clicked!");
});
</script>
</body>
</html>
In the example above, when you click the button, you will first see the alert Child clicked!
, but the event will bubble up and trigger the Parent clicked!
message as well. This is a typical example of Event Bubbling.
What is Event Delegation?
Event Delegation is the technique of handling events by attaching the event listener to a parent element instead of individual child elements. This approach increases efficiency and results in cleaner code, as you don’t need to attach event listeners to each child element separately, but rather to a common parent.
Event Delegation is especially useful when dealing with dynamically created elements. If an element is added to the DOM later, it will automatically be handled by the parent’s event listener.
How Does Event Delegation Work?
Event Delegation works by adding an event listener to a parent element and then using event.target
to determine which child element triggered the event.
Example: Event Delegation
<!DOCTYPE html>
<html lang="tr">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Event Delegation</title>
</head>
<body>
<div id="parent">
<button class="child">Button 1</button>
<button class="child">Button 2</button>
<button class="child">Button 3</button>
</div>
<script>
// Event listener on Parent div
document.getElementById("parent").addEventListener("click", function(event) {
// Checking if the clicked element is a button with class 'child'
if(event.target && event.target.matches("button.child")) {
alert(event.target.textContent + " clicked!");
}
});
</script>
</body>
</html>
In this example, we attach the event listener to the parent
element. When a button
with the class child
is clicked, the corresponding message will be displayed. This is a practical example of Event Delegation.
Differences Between Event Bubbling and Event Delegation
- Event Bubbling: The event propagates upwards from the target element to its parent elements.
- Event Delegation: The event listener is attached to a parent element, and the target element is determined using
event.target
.
Use Cases:
- Event Bubbling is typically used when you need to catch events as they propagate upwards and in situations where you need to attach event listeners to multiple elements.
- Event Delegation is preferred for handling dynamically created elements and when performance is a concern, as it avoids attaching event listeners to each individual element.
When Should We Use Them?
- Event Bubbling: It's useful in static DOM structures and for simpler scenarios.
- Event Delegation: It’s more efficient for dynamic content (elements added or removed from the DOM) and in larger applications.
Using Event Bubbling and Event Delegation
To control Event Bubbling, you can use the event.stopPropagation()
method. This stops the event from bubbling further.
Example: Stopping Event Bubbling
<script>
document.getElementById("child").addEventListener("click", function(event) {
alert("Child clicked!");
event.stopPropagation(); // Prevent bubbling
});
document.getElementById("parent").addEventListener("click", function() {
alert("Parent clicked!");
});
</script>
In this example, event.stopPropagation()
prevents the event from bubbling up to the parent element, so the Parent clicked!
alert won’t be shown when clicking the child element.
Conclusion
Event Bubbling and Event Delegation are powerful techniques for managing events in JavaScript. Understanding these concepts allows you to work more efficiently with DOM events and create more optimized and maintainable JavaScript code.
- Event Bubbling allows you to capture events as they propagate upwards, but care should be taken to avoid unwanted behavior.
- Event Delegation is an excellent technique for handling dynamically generated content and improves performance, especially in large applications.
By applying both techniques correctly, you can write more efficient and scalable JavaScript code.
We hope this blog post helps you understand Event Bubbling and Event Delegation in JavaScript, and how to implement them in your projects!
Leave a Comment