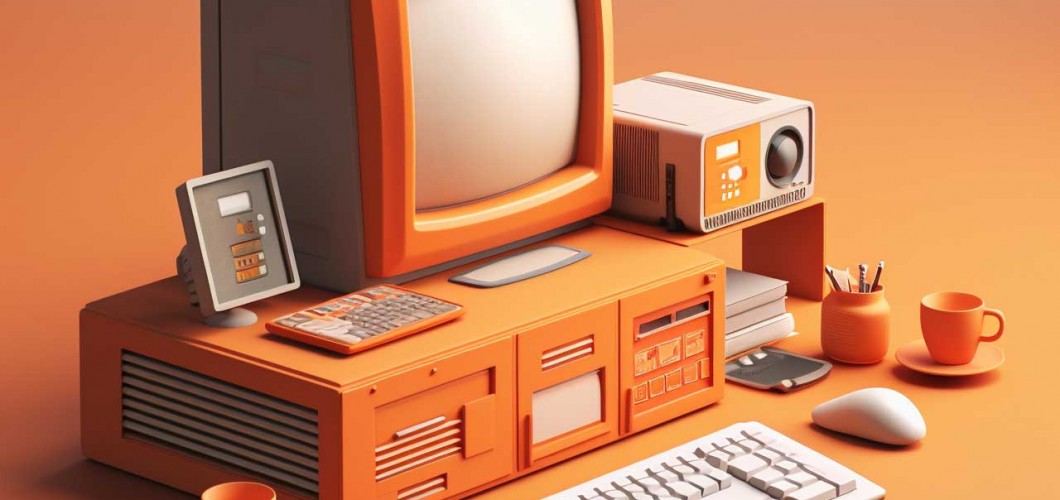
JavaScript Event Listeners: The Best Ways to Manage Events
JavaScript is one of the most important tools when creating interactive web pages, and event handling is a key part of this interaction. User actions such as mouse clicks, key presses, form submissions, or page loads allow users to interact with your website. Managing these events properly plays a critical role in providing a good user experience.
In this article, we will explore what JavaScript Event Listeners are, how they are used, different methods for handling them, and best practices with detailed explanations and examples.
1. What is an Event Listener?
In JavaScript, an "Event Listener" is a mechanism that triggers a specific function (callback function) when a particular event occurs. These event listeners, attached to elements on a webpage, listen for user interactions (such as clicks, scrolls, keyboard inputs) and trigger the function you've defined when those interactions happen.
2. Using Event Listeners
To add an Event Listener, we use the addEventListener()
method. This method attaches an event listener to a specified element and triggers the function when the event occurs.
Basic Usage:
element.addEventListener('event', callback);
element
: The HTML element you want to listen to.'event'
: The type of event you want to listen for (e.g.,'click'
,'keypress'
,'load'
).callback
: The function that will be triggered when the event happens.
3. Types of Events
JavaScript has a variety of event types to listen for. These events help capture user interactions and browser behaviors. Below are some of the common event types:
a. click
Event
Triggered when a user clicks on an element.
const button = document.getElementById('myButton');
button.addEventListener('click', () => {
alert('Button clicked!');
});
b. keypress
Event
Triggered when a user presses a key on the keyboard.
document.addEventListener('keypress', (event) => {
console.log(`Key pressed: ${event.key}`);
});
c. mouseenter
and mouseleave
Events
Triggered when the mouse pointer enters (mouseenter
) or leaves (mouseleave
) an element.
const box = document.getElementById('box');
box.addEventListener('mouseenter', () => {
box.style.backgroundColor = 'lightblue';
});
box.addEventListener('mouseleave', () => {
box.style.backgroundColor = 'white';
});
d. input
Event
Triggered when the value of an input element changes.
const input = document.getElementById('myInput');
input.addEventListener('input', (event) => {
console.log(`Input value: ${event.target.value}`);
});
4. Parameters of addEventListener
When adding an event listener, there are several important parameters:
- Event Type: The type of event you want to listen for.
- Callback (Callback Function): The function that will be executed when the event occurs.
- Options (Optional Parameters): These are additional settings that define how the event will be handled. This parameter is optional.
Example:
element.addEventListener('click', callback, { capture: true, once: true });
capture
: If set totrue
, the event will be triggered during the capture phase (before it bubbles up).once
: If set totrue
, the event will only be triggered once and will be automatically removed afterward.
5. Tips for Working with Event Listeners
a. Using removeEventListener
To remove an event listener, use the removeEventListener
method. This prevents the function from being executed again for the event.
const button = document.getElementById('myButton');
const handleClick = () => {
alert('Button clicked!');
};
button.addEventListener('click', handleClick);
button.removeEventListener('click', handleClick);
b. Event Bubbling and Capturing
In JavaScript, events propagate in two phases: bubbling and capturing. Understanding these two phases helps in managing events more effectively.
- Bubbling: The event propagates from the innermost element outward (this is the default behavior).
- Capturing: The event propagates from the outermost element inward.
Understanding the differences between bubbling and capturing is crucial for structuring your event listeners correctly.
c. Event Delegation
Event delegation is a technique where you add a single event listener to a parent element to manage events for its child elements. This is especially useful for performance when there are many elements to handle.
Example:
document.getElementById('parent').addEventListener('click', (event) => {
if (event.target && event.target.matches('button.className')) {
alert('Button clicked!');
}
});
In this example, the event listener is added to the parent element, and it only triggers the callback if the target is a specific button.
6. Best Practices
a. Avoid Overusing Event Listeners
Adding unnecessary event listeners for every element can consume memory and slow down performance. Instead, consider using event delegation to add a single listener to a parent element for all child elements.
b. Manage Event Listeners
As event listeners can accumulate on the page, it's important to remove them when they are no longer needed. This prevents memory leaks and improves performance.
c. Use the once
Option for One-Time Events
For events that only need to happen once (e.g., a modal opening), use the once: true
option to ensure the event listener is automatically removed after the first trigger.
7. Summary
JavaScript Event Listeners are essential for building interactive web applications. Knowing how to handle events properly, understanding the different phases of event propagation (bubbling and capturing), using event delegation, and managing event listeners efficiently are all important skills to have for creating high-quality, responsive web pages.
By mastering event listeners and understanding how they can be optimized, you can create more efficient, faster, and user-friendly web experiences.
Leave a Comment