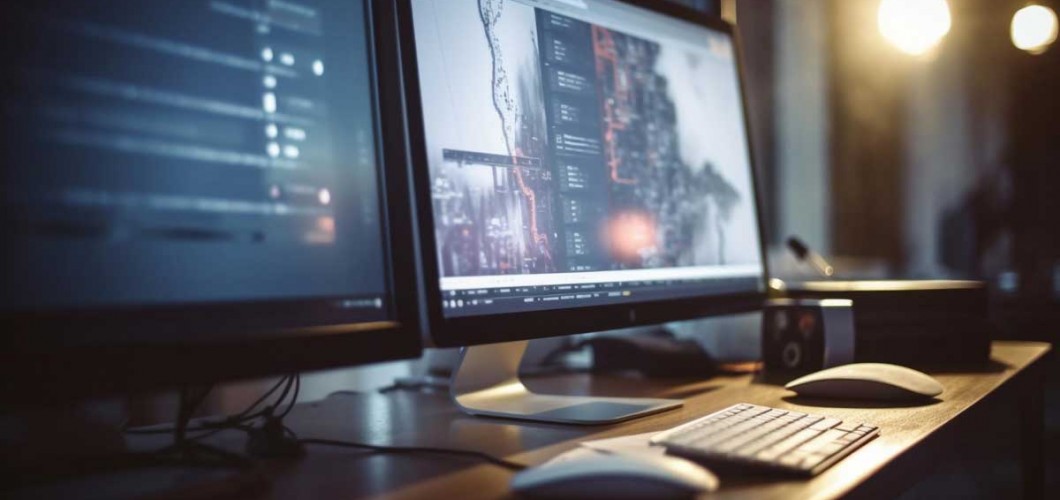
JavaScript Functions: Basics and Advanced Usage
JavaScript is one of the most commonly used programming languages for web development. One of the powerful features of JavaScript is its functions and their flexible usage. In this article, we will explore JavaScript functions from the basics to advanced levels, and review various examples of how functions can be used in different ways.
1. Basic Usage of JavaScript Functions
Functions are blocks of code designed to perform a specific task. In JavaScript, functions can be defined in various ways. The most basic method of defining a function is through a function declaration.
Defining a Function
To define a function, the function
keyword is used. Here's a simple example of a function:
function greet() {
console.log("Hello, world!");
}
greet(); // Output: Hello, world!
In this example, we defined a function called greet
and called it. The function simply prints a message using console.log
.
Function Parameters
Functions can accept parameters. Parameters are used to pass data to a function from the outside.
function greet(name) {
console.log("Hello, " + name + "!");
}
greet("Ahmet"); // Output: Hello, Ahmet!
greet("Zeynep"); // Output: Hello, Zeynep!
In the example above, the greet
function takes a parameter and uses it within the message.
Returning a Value from a Function
A function can return a value. To return a value, the return
keyword is used.
function add(a, b) {
return a + b;
}
let result = add(5, 3);
console.log(result); // Output: 8
In this example, the add
function adds two numbers and returns the result.
2. Function Expressions
Functions can also be defined as expressions and assigned to variables. This is done using function expressions.
const multiply = function(a, b) {
return a * b;
};
console.log(multiply(4, 5)); // Output: 20
In this approach, the function is an anonymous function that is assigned to a variable. This function can only be called after it has been defined.
3. Arrow Functions
Arrow functions, introduced in ES6, offer a more concise syntax for defining functions. They are especially useful for single-line functions.
const add = (a, b) => a + b;
console.log(add(5, 10)); // Output: 15
If a function takes only one parameter, the parentheses can be omitted:
const square = x => x * x;
console.log(square(4)); // Output: 16
Arrow functions behave differently than traditional functions when it comes to the this
keyword. Arrow functions do not bind their own this
, they inherit it from the surrounding context.
4. Advanced Function Usage
JavaScript functions are powerful not just in basic usage, but also in advanced scenarios. Let's now explore some advanced ways of using functions.
Higher-Order Functions
Higher-order functions are functions that can take other functions as parameters or return functions.
function performOperation(operation, x, y) {
return operation(x, y);
}
function add(a, b) {
return a + b;
}
function multiply(a, b) {
return a * b;
}
console.log(performOperation(add, 5, 3)); // Output: 8
console.log(performOperation(multiply, 5, 3)); // Output: 15
In this example, the performOperation
function takes other functions (add
and multiply
) as arguments and calls them.
Closures
In JavaScript, a function can be defined inside another function, and the inner function can access the parameters and variables of the outer function. This is known as a "closure."
function outerFunction(x) {
return function innerFunction(y) {
return x + y;
};
}
const add5 = outerFunction(5);
console.log(add5(3)); // Output: 8
console.log(add5(10)); // Output: 15
In this example, the outerFunction
returns an innerFunction
, and the inner function has access to the outer function's parameter, x
. This creates a closure where x
is preserved even after the outerFunction
has finished executing.
Default Parameters in Functions
With ES6, it's possible to assign default values to function parameters. If a parameter is not provided, the default value is used.
function greet(name = "Guest") {
console.log("Hello, " + name + "!");
}
greet(); // Output: Hello, Guest!
greet("Ahmet"); // Output: Hello, Ahmet!
In this example, if no value is passed for the name
parameter, it defaults to "Guest"
.
Rest Parameters and Spread Operator
Rest parameters allow a function to accept an arbitrary number of arguments. They are denoted by ...
.
function sum(...numbers) {
return numbers.reduce((total, num) => total + num, 0);
}
console.log(sum(1, 2, 3)); // Output: 6
console.log(sum(10, 20, 30, 40)); // Output: 100
Here, ...numbers
collects all the passed arguments into an array, and we can then perform operations on them, such as summing them up.
Using this
with Functions
In JavaScript, the value of this
depends on how a function is called. However, with arrow functions, this
is lexically bound (it inherits from the surrounding context).
const obj = {
name: "JavaScript",
greet: function() {
console.log("Hello, " + this.name);
}
};
obj.greet(); // Output: Hello, JavaScript
If we had used an arrow function, the behavior of this
would be different:
const obj = {
name: "JavaScript",
greet: () => {
console.log("Hello, " + this.name); // `this` refers to the global object
}
};
obj.greet(); // Output: Hello, undefined
5. Conclusion
JavaScript functions can be used in a wide variety of ways, from basic usage to more advanced scenarios. Starting from basic function declarations, parameters, and return values, you can explore more complex features like function expressions, arrow functions, higher-order functions, closures, default parameters, and this
usage.
By mastering functions at all levels, you can write more effective and efficient JavaScript code. With the concepts we've covered in this article, you should have a deeper understanding of how to work with functions in JavaScript, making your coding practices more powerful and flexible.
Leave a Comment