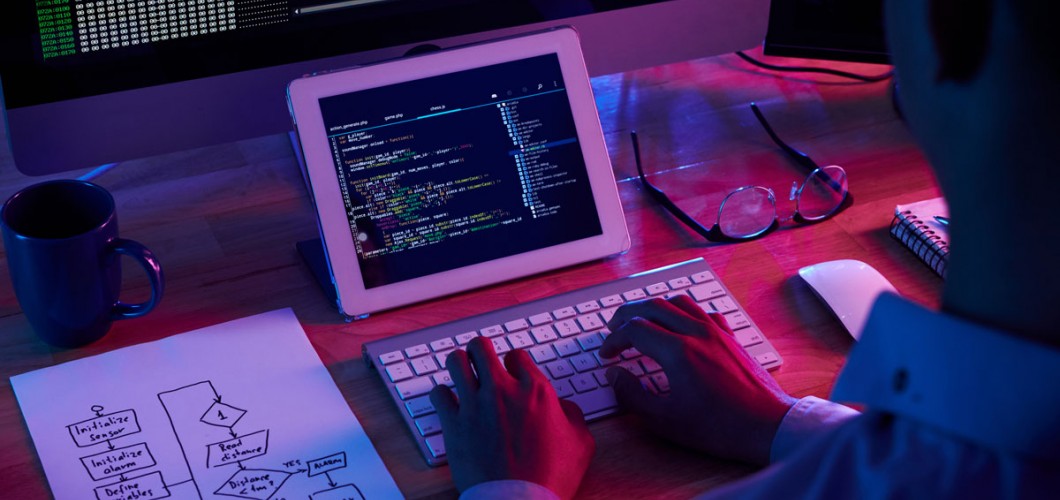
JavaScript Object and Array Methods: map
, filter
, reduce
JavaScript is one of the most widely used languages in modern software development. With the powerful methods it provides, we can process data more effectively. In this article, we will take an in-depth look at three important and frequently used array methods in JavaScript: map
, filter
, and reduce
. These methods enable us to write cleaner and more readable code by embracing functional programming principles.
1. map
Method
Definition
The map
method creates a new array populated with the results of calling a provided function on every element in the calling array. This method does not modify the original array; instead, it creates a new array with the results.
Usage
let numbers = [1, 2, 3, 4, 5];
let squares = numbers.map(num => num * num);
console.log(squares); // [1, 4, 9, 16, 25]
Explanation
- The
map
method executes the provided callback function for each element in the array. - Here,
num => num * num
squares each element.
Real-World Example
Let's apply a 10% discount on each product in a shopping cart:
let products = [
{ name: 'Phone', price: 3000 },
{ name: 'Laptop', price: 5000 },
{ name: 'Tablet', price: 2000 }
];
let discountedProducts = products.map(product => ({
name: product.name,
price: product.price * 0.9
}));
console.log(discountedProducts);
// [{name: 'Phone', price: 2700}, {name: 'Laptop', price: 4500}, {name: 'Tablet', price: 1800}]
In this example, we applied a 10% discount to each product's price and created a new array with the discounted prices.
2. filter
Method
Definition
The filter
method creates a new array with all elements that pass the test implemented by the provided function. It filters out elements that do not meet the specified condition, returning only those that satisfy it. The original array is not modified.
Usage
let numbers = [1, 2, 3, 4, 5, 6];
let evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers); // [2, 4, 6]
Explanation
- The
filter
method evaluates the provided condition for each element in the array. - Here,
num => num % 2 === 0
filters out even numbers.
Real-World Example
Let’s list only the products that are in stock from an e-commerce store:
let products = [
{ name: 'Phone', inStock: true },
{ name: 'Laptop', inStock: false },
{ name: 'Tablet', inStock: true }
];
let inStockProducts = products.filter(product => product.inStock);
console.log(inStockProducts);
// [{name: 'Phone', inStock: true}, {name: 'Tablet', inStock: true}]
In this example, we filter the products with the inStock
property set to true
, listing only the available products.
3. reduce
Method
Definition
The reduce
method applies a function to each element in the array (from left to right) to reduce it to a single value. It accumulates the result of each operation into a single return value. This method is typically used for operations like summing, multiplying, etc.
Usage
let numbers = [1, 2, 3, 4, 5];
let total = numbers.reduce((sum, num) => sum + num, 0);
console.log(total); // 15
Explanation
- The
reduce
method takes two parameters: a callback function and an initial value. - The callback function has two parameters: the accumulated value (
sum
) and the current element (num
). - Initially, the accumulated value (
sum
) is set to0
, and each element is added to it.
Real-World Example
Let's calculate the total price of all products in a shopping cart:
let products = [
{ name: 'Phone', price: 3000 },
{ name: 'Laptop', price: 5000 },
{ name: 'Tablet', price: 2000 }
];
let totalPrice = products.reduce((sum, product) => sum + product.price, 0);
console.log(totalPrice); // 10000
In this example, we sum up the prices of all products to get the total price of the cart.
Differences Between map
, filter
, and reduce
map
: Transforms the array and applies the given function to each element, returning a new array without modifying the original.filter
: Filters out elements that don’t meet the specified condition and returns a new array of elements that pass the condition.reduce
: Reduces the array to a single value by applying the specified operation, typically used for summing, multiplying, or accumulating values.
Conclusion
The map
, filter
, and reduce
methods in JavaScript are powerful tools that allow us to manipulate and process arrays efficiently. By using these methods, we can write shorter, cleaner, and more understandable code. Especially for those embracing functional programming, these methods are extremely useful. Using them effectively in software development can help you create more efficient and maintainable projects.
Leave a Comment