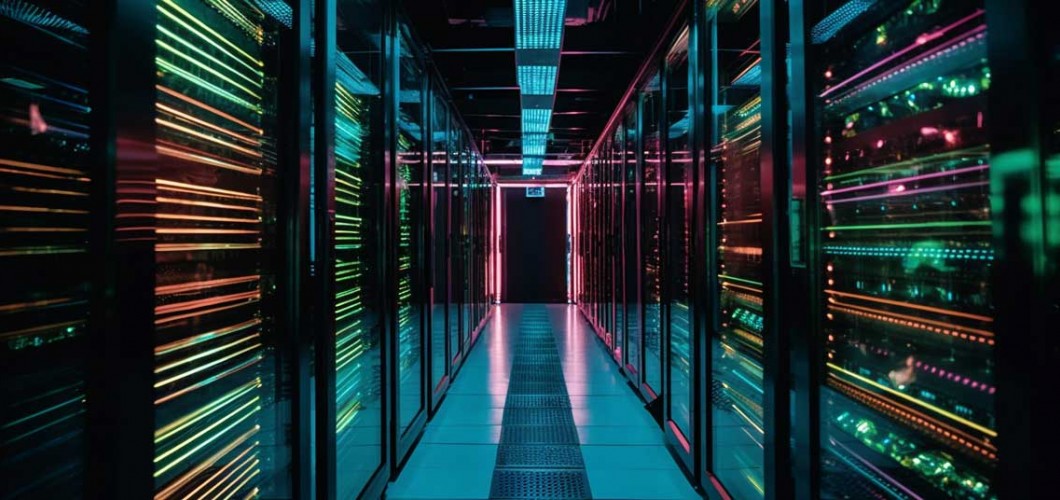
JavaScript Variables and Data Types: In-Depth Review
JavaScript, as a dynamic programming language, offers flexibility and power when it comes to variables and data types. In this article, we will explore JavaScript variables, data types, and how each of them works in detail.
1. Variables in JavaScript
Variables are fundamental building blocks used to store data in our programs. In JavaScript, variables can be defined in three ways: var
, let
, and const
.
a. Defining Variables with var
var
is the original way to declare variables in JavaScript and was commonly used before ES6. Variables declared with var
have function scope or global scope. This means variables declared inside a function are accessible only within that function, while those declared outside are accessible globally.
Hoisting: Variables declared with var
are hoisted, meaning they are considered declared even before their declaration code is executed. However, if the value hasn't been assigned yet, it will be undefined
.
console.log(a); // undefined
var a = 5;
console.log(a); // 5
b. Defining Variables with let
Introduced with ES6, let
is a more modern alternative to var
and has block scope. Variables declared with let
are accessible only within the block they are defined in.
Hoisting: While let
variables are hoisted, they must be declared before use, or a "Temporal Dead Zone (TDZ)" error will occur.
let b = 10;
console.log(b); // 10
// Hoisting error
console.log(c); // ReferenceError: Cannot access 'c' before initialization
let c = 20;
c. Defining Variables with const
const
declares a constant variable, meaning its value cannot be reassigned after initial assignment. However, if a reference type like an object or array is assigned, its content can be modified, but the reference itself cannot be reassigned.
Hoisting: const
is also hoisted but must be declared before use.
const d = 30;
console.log(d); // 30
// Cannot reassign
// d = 40; // TypeError: Assignment to constant variable.
const e = [1, 2, 3];
e.push(4); // This is valid as the array's contents can change.
console.log(e); // [1, 2, 3, 4]
2. JavaScript Data Types
Data types in JavaScript define the kind of data that can be assigned to variables. They are categorized into two main types: Primitive Data Types and Reference Data Types.
a. Primitive Data Types
Primitive types store actual values directly. JavaScript's primitive types include:
- Number: Represents numerical values, including integers and floating-point numbers.
let num = 42;
let decimal = 3.14;
- String: Represents textual data, which can be enclosed in single or double quotes.
let name = "Ali";
let greeting = 'Hello, world!';
- Boolean: Represents logical values
true
orfalse
.
let isActive = true;
let isValid = false;
- Undefined: A variable that has been declared but not assigned a value.
let a;
console.log(a); // undefined
- Null: Explicitly represents "no value" or "empty value".
let user = null;
- Symbol (Introduced in ES6): Represents a unique and immutable value.
let sym = Symbol('description');
b. Reference Data Types
Reference types store a reference to the value rather than the value itself. They include:
- Object: Represents key-value pairs. Objects are one of the most commonly used types in JavaScript for storing complex data structures.
let person = {
name: "Ali",
age: 25,
greet: function() {
console.log("Hello!");
}
};
- Array: Represents ordered collections and can store multiple data types.
let numbers = [1, 2, 3, 4, 5];
- Function: Functions are also considered a type in JavaScript.
function greet() {
console.log("Hello!");
}
3. Differences and Conversions Between Data Types
JavaScript allows conversions between data types, known as Type Coercion. There are two types:
- Implicit Type Coercion: JavaScript automatically converts types when needed.
let result = "5" + 1; // "51" (string)
- Explicit Type Conversion: Developers manually convert data types using functions like
String()
,Number()
, andBoolean()
.
let num = Number("10"); // 10 (number)
let str = String(123); // "123" (string)
let bool = Boolean(0); // false
4. Conclusion
Understanding variables and data types in JavaScript highlights the language's flexibility and strength. Knowing when to use the right data types and variable declarations helps write efficient, error-free code. Proper selection of data types and variable declaration methods improves performance and reduces bugs in JavaScript applications.
Leave a Comment