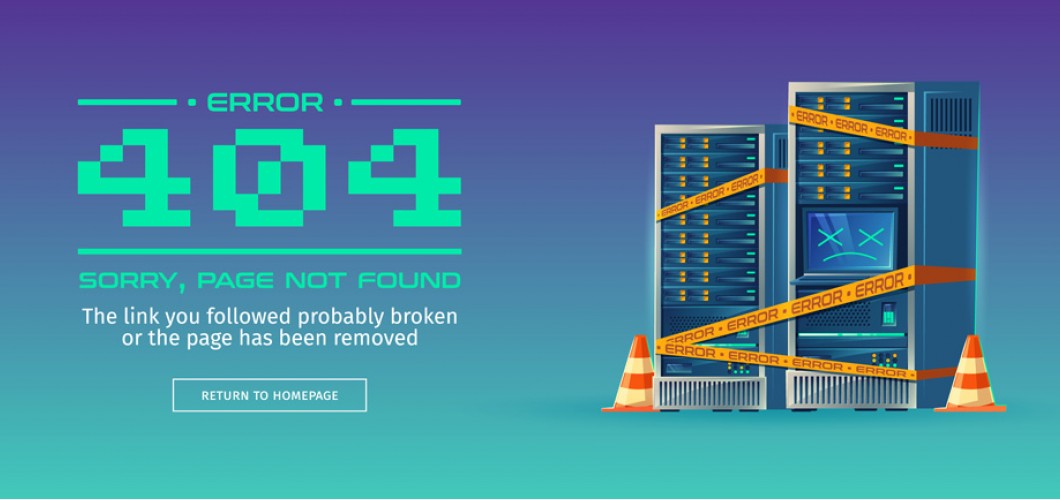
SEO Optimization and Server-Side Rendering (SSR) in JavaScript: A Comprehensive Guide
The performance of web applications, user experience, and visibility in search engines are some of the most important factors in SEO (Search Engine Optimization). However, modern JavaScript-based applications can face certain challenges in terms of SEO. Fortunately, techniques like Server-Side Rendering (SSR) are key to creating SEO-friendly web applications. In this article, we will explore how SEO optimization in JavaScript works, why it’s important, and how to implement it using Server-Side Rendering (SSR).
1. What is SEO and Why is it Important?
SEO is the process of optimizing a website or application to rank higher in search engine results. The main goal of SEO is to ensure that search engines understand a web page correctly and that users can easily find the page in search results. Proper indexing and crawling of your website by search engines is one of the most effective ways to increase organic traffic.
Main Objectives of SEO:
- Search Engine Visibility: Users can find your website more easily with higher rankings and visibility on search engines.
- Fast Access and Performance: Page speed and accessibility are crucial factors for SEO.
- Mobile Compatibility: Mobile-friendly pages directly affect SEO.
- Content Optimization: Well-optimized content improves both user experience and SEO.
2. JavaScript and SEO: Challenges and Solutions
Modern web applications are often developed with JavaScript. However, JavaScript-based applications can have SEO issues. This is because search engines sometimes struggle to properly index dynamically loaded content. JavaScript’s dynamic nature means that page content changes as it loads, which can prevent search engines from understanding the content.
SEO Challenges in JavaScript:
- Search Engine Crawlers: Search engine bots may not process JavaScript code effectively to load content dynamically.
- Dynamic Content Loading: Content loaded via JavaScript after the page loads makes it harder for search engines to crawl the content.
- URL Parameters and Structure: Complex URL structures can prevent search engines from indexing pages correctly.
Solution: Server-Side Rendering (SSR)
SSR refers to the technique of generating the page content on the server and sending fully rendered HTML to the browser. This allows search engines to directly access the content and index it properly, making JavaScript-based applications SEO-friendly. With SSR, the application is rendered on the server, and search engine bots can easily index dynamic content.
3. What is Server-Side Rendering (SSR)?
Server-Side Rendering (SSR) refers to the process of rendering the web page content on the server and sending it to the client (browser) as fully rendered HTML. In traditional Client-Side Rendering (CSR), the HTML structure and content are dynamically generated by JavaScript in the browser. With SSR, the page is pre-rendered on the server, and HTML content is sent to the browser, making it easier for search engine bots to crawl and index the content.
How SSR Improves SEO:
- Search Engine Accessibility: SSR allows search engine bots to access and index dynamic content correctly.
- Faster Loading: Users receive rendered HTML content quickly, improving user experience and boosting SEO.
- Mobile Compatibility: SSR provides a faster and smoother experience on mobile devices, which also improves SEO.
4. Using SSR in JavaScript Applications
SSR is commonly used in JavaScript applications, particularly in React.js applications. Popular frameworks like Next.js and Nuxt.js can be used to implement SSR.
SSR with React.js and Next.js:
Next.js is a popular framework for implementing SSR in React applications. With Next.js, SSR allows the server to handle page rendering and sends the pre-rendered page to the client quickly.
SSR Example with Next.js:
// pages/index.js
import React from 'react';
// Fetching data and rendering it on the server before sending to the browser
const HomePage = ({ data }) => {
return (
<div>
<h1>Page Rendered with SSR</h1>
<p>{data}</p>
</div>
);
};
// getServerSideProps fetches the data on the server before rendering the page
export async function getServerSideProps() {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return {
props: { data }, // Sending the data to the page
};
}
export default HomePage;
In this example, Next.js’ getServerSideProps
function fetches the data on the server before rendering the page and sends it to the browser. This ensures that search engines get the content directly.
SSR with Nuxt.js:
Nuxt.js is a framework for implementing SSR in Vue.js applications. Nuxt.js also renders pages on the server, ensuring SEO-friendly content.
SSR Example with Nuxt.js:
// pages/index.vue
<template>
<div>
<h1>Page Rendered with SSR</h1>
<p>{{ data }}</p>
</div>
</template>
<script>
export default {
async asyncData({ params }) {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return { data };
}
}
</script>
In this example, Nuxt.js’s asyncData
function fetches the data and pre-renders it on the server before sending it to the browser.
5. Additional Tips for SSR and SEO Optimization
Here are some other important tips for optimizing SEO with SSR:
- Dynamically Update Meta Tags: SSR can be used to dynamically update page titles and meta descriptions. This ensures that each page has specific meta data for search engines.
- Performance Optimization: Fast page loading is a key factor in SEO. SSR increases initial render speed, improving both performance and SEO.
- Social Sharing Optimization: Use proper
Open Graph
andTwitter Card
meta tags to ensure optimal social media sharing. - Canonical URL Usage: If you have multiple versions of a page, use the correct canonical URL tag to avoid duplicate content issues.
Conclusion
SEO optimization in JavaScript-based applications can be challenging, especially with dynamic content and JavaScript’s behavior. However, Server-Side Rendering (SSR) provides a solution to overcome these challenges. SSR allows search engines to directly index pre-rendered content, making JavaScript-based applications SEO-friendly. Modern frameworks like React.js and Next.js offer easy ways to implement SSR.
By using SSR, you can improve SEO, enhance user experience, and increase rankings in search engine results.
Leave a Comment