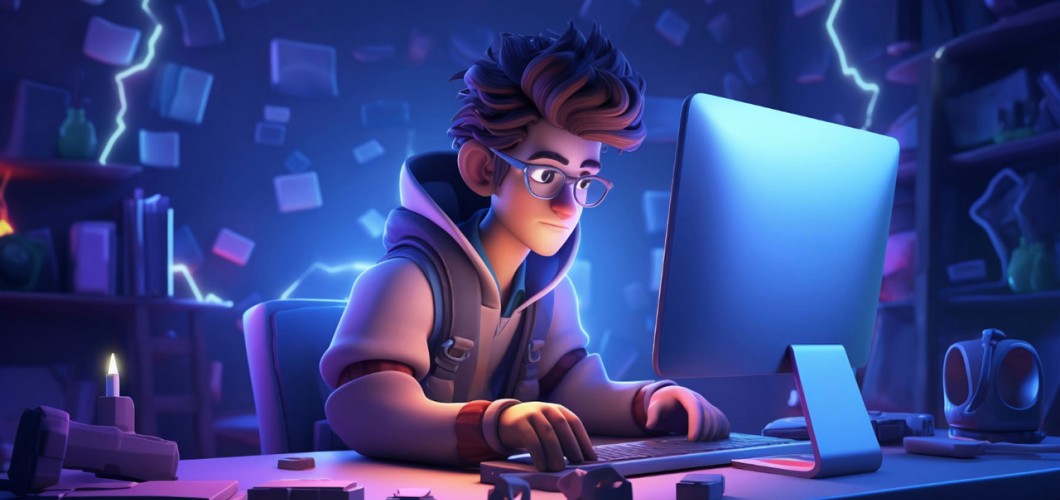
Worker Threads and Parallel Programming in JavaScript
INTRODUCTION
JavaScript is designed as a single-threaded and single-core programming language, meaning it processes code sequentially. However, modern applications often involve complex, computation-heavy tasks, which can lead to bottlenecks in a single-threaded environment. This is where Worker Threads come into play.
In this article, we will explore Worker Threads in JavaScript and parallel programming in detail, supported by examples, and discuss the benefits of using this technology.
What Are Worker Threads?
Node.js supports parallel programming through its Worker Threads module. Worker Threads enable you to perform CPU-intensive tasks without blocking the main thread.
Key Features of Worker Threads:
- Parallel Processing: Create worker threads to handle CPU-heavy tasks concurrently.
- Message-Based Communication: Worker Threads communicate with the main thread using a message-passing mechanism.
- Improved Performance: Prevents blocking operations, ensuring smoother performance.
How to Use the Worker Threads Module
To use the Worker Threads module, import it as follows:
const { Worker, isMainThread, parentPort } = require('worker_threads');
Basic Example of Worker Threads
Main File (main.js)
const { Worker } = require('worker_threads');
function runService(workerData) {
return new Promise((resolve, reject) => {
const worker = new Worker('./worker.js', { workerData });
worker.on('message', resolve);
worker.on('error', reject);
worker.on('exit', (code) => {
if (code !== 0)
reject(new Error(`Worker stopped with exit code ${code}`));
});
});
}
runService('Hello, Worker!').then(result => console.log(result)).catch(err => console.error(err));
Worker File (worker.js)
const { parentPort, workerData } = require('worker_threads');
// Data processing
taskResult = workerData.toUpperCase();
// Send result back to the main thread
parentPort.postMessage(taskResult);
Explanation:
- The
main.js
file creates a Worker and instructs it to run theworker.js
file. - The
worker.js
file processes the incoming data and sends the result back to the main thread.
Parallel Processing Performance Example
Let's observe how Worker Threads can speed up computation-intensive tasks.
CPU-Intensive Computation (heavyTask.js)
const { parentPort, workerData } = require('worker_threads');
function heavyComputation(n) {
let count = 0;
for (let i = 0; i < n; i++) {
count += i;
}
return count;
}
const result = heavyComputation(workerData);
parentPort.postMessage(result);
Main File (main.js)
const { Worker } = require('worker_threads');
console.time('performance');
const worker = new Worker('./heavyTask.js', { workerData: 1e8 });
worker.on('message', result => {
console.log(`Result: ${result}`);
console.timeEnd('performance');
});
worker.on('error', console.error);
Expected Output:
Result: 4999999950000000
performance: 100ms
This approach allows computations to be processed without blocking the main thread.
Message Passing with Worker Threads
Worker Threads use parentPort.postMessage and on('message') for communication.
// worker.js
const { parentPort } = require('worker_threads');
parentPort.on('message', (msg) => {
parentPort.postMessage(`Received: ${msg}`);
});
// main.js
const { Worker } = require('worker_threads');
const worker = new Worker('./worker.js');
worker.on('message', (msg) => console.log(msg));
worker.postMessage('Hello, Worker!');
Advantages of Worker Threads
- Performance Boost: Efficiently handles CPU-intensive tasks.
- Prevents Main Thread Blocking: Keeps the main thread responsive.
- Secure Message Passing: Ensures safe data processing.
Conclusion
In JavaScript, Worker Threads are one of the most effective ways to achieve parallel programming. This method allows you to perform heavy tasks without blocking the main thread, enhancing application performance.
The Worker Threads module is a significant advantage for developers working on Node.js projects requiring concurrency. For complex scenarios and multi-data processing, this approach is invaluable.
Leave a Comment