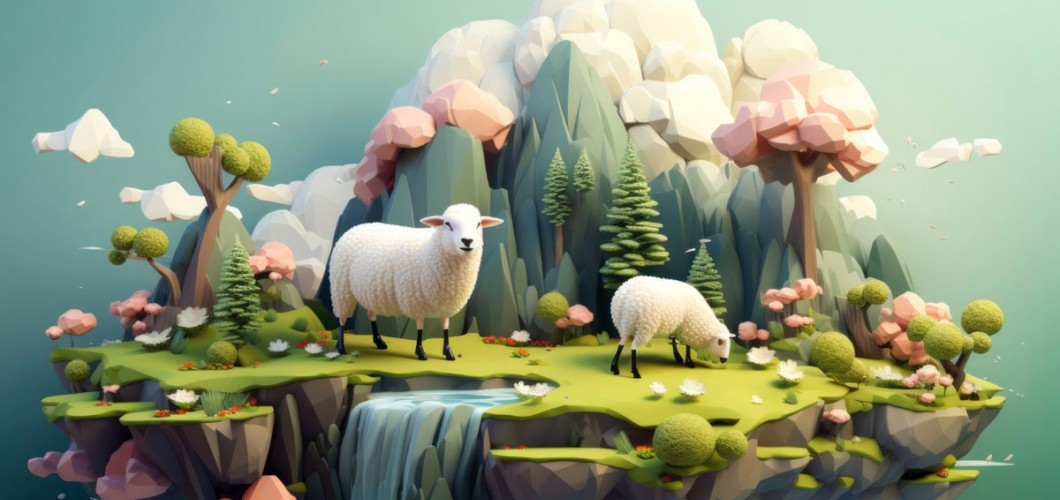
3D Modeling with Three.js in JavaScript: A Step-by-Step Guide
Three.js is one of the most popular libraries for creating 3D graphics using JavaScript. Three.js allows you to create dynamic and interactive graphics for web applications. In this post, we will provide a comprehensive guide on how to perform 3D modeling with Three.js.
1. What is Three.js?
Three.js is an open-source JavaScript library used to create 3D graphics. Working on WebGL, Three.js enables you to create real-time 3D graphics and animations directly in the browser. Three.js provides numerous tools to make 3D graphics visually stunning and interactive.
With Three.js, you can:
- Create 3D models,
- Add lighting, shadow, and material effects,
- Create 3D animations and design interactive scenes.
2. Tools Required for 3D Modeling with Three.js
2.1. Installing the Three.js Library
First, we need to include the Three.js library in our project. We can either use npm to install the library or include it directly through a CDN.
Loading Three.js via CDN:
<script src="https://cdn.jsdelivr.net/npm/three@0.130.1/build/three.min.js"></script>
Installing via npm:
npm install three
2.2. Basic HTML Structure
To start with 3D modeling using Three.js, we need a simple HTML structure. Here's an example HTML file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Three.js 3D Modeling</title>
<style>
body { margin: 0; }
canvas { display: block; }
</style>
</head>
<body>
<script src="https://cdn.jsdelivr.net/npm/three@0.130.1/build/three.min.js"></script>
<script src="app.js"></script>
</body>
</html>
3. Creating a Simple 3D Scene with Three.js
Let's create a simple 3D scene with Three.js. We will add a camera, a scene, a light, and a cube.
app.js:
// 1. Create Scene
const scene = new THREE.Scene();
// 2. Create Camera
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
// 3. Create WebGL Renderer
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// 4. Create Cube Geometry and Material
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
// 5. Add Cube to Scene
scene.add(cube);
// 6. Set Camera Position
camera.position.z = 5;
// 7. Animation Loop
function animate() {
requestAnimationFrame(animate);
// Rotate the Cube
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
// Render the Scene
renderer.render(scene, camera);
}
// Start Animation
animate();
This simple example creates a cube and starts an animation that rotates it.
4. Three.js 3D Modeling: Geometry and Materials
4.1. Types of Geometries
Three.js provides various geometries to create different 3D shapes, such as:
- BoxGeometry: Cube
- SphereGeometry: Sphere
- CylinderGeometry: Cylinder
- TorusGeometry: Torus
- PlaneGeometry: Plane
Each geometry is a subclass of the THREE.Geometry
class, and you can adjust the shape's dimensions by passing parameters.
4.2. Types of Materials
You can add different materials to your 3D models in Three.js. For example:
- MeshBasicMaterial: Flat color, without lighting effects
- MeshLambertMaterial: Light-affected, matte surface
- MeshPhongMaterial: Light and reflection effects, shiny surface
5. Three.js 3D Modeling: Lighting and Camera
5.1. Lighting
Lighting is crucial in Three.js. It affects the visibility of objects in the scene and gives them a realistic look. Here are the most common light types:
- AmbientLight: General, soft light
- DirectionalLight: Light coming from a specific direction, like sunlight
- PointLight: A light source from a point
Example Lighting:
// AmbientLight
const ambientLight = new THREE.AmbientLight(0x404040); // Soft white light
scene.add(ambientLight);
// DirectionalLight
const directionalLight = new THREE.DirectionalLight(0xffffff, 1);
directionalLight.position.set(1, 1, 1).normalize();
scene.add(directionalLight);
5.2. Camera Usage
The camera in Three.js defines how the scene will be viewed. Typically, two types of cameras are used:
- PerspectiveCamera: Provides a realistic perspective (distances shrink as they go further away).
- OrthographicCamera: Provides a parallel view (distances remain the same).
Camera and Perspective:
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
camera.position.z = 5;
6. Advanced Three.js Features
6.1. Importing 3D Models
You can import external 3D models into Three.js using file formats like .obj
, .fbx
, .gltf
. These files can be loaded into the project using the appropriate loaders in Three.js.
For example, to load a .gltf
file:
const loader = new THREE.GLTFLoader();
loader.load('model.gltf', function(gltf) {
scene.add(gltf.scene);
});
6.2. 3D Animations
Three.js also makes it easy to create animations. You can animate objects using keyframe animations or bone-based animations for characters and other objects.
7. Conclusion
Three.js is a powerful tool that can take your web projects to the next level with 3D modeling and graphics. In this guide, we explored how to create a basic Three.js scene, work with geometry, materials, lighting, camera, and animations. Thanks to its extensive features, Three.js offers numerous possibilities for 3D modeling and visualization projects.
Leave a Comment