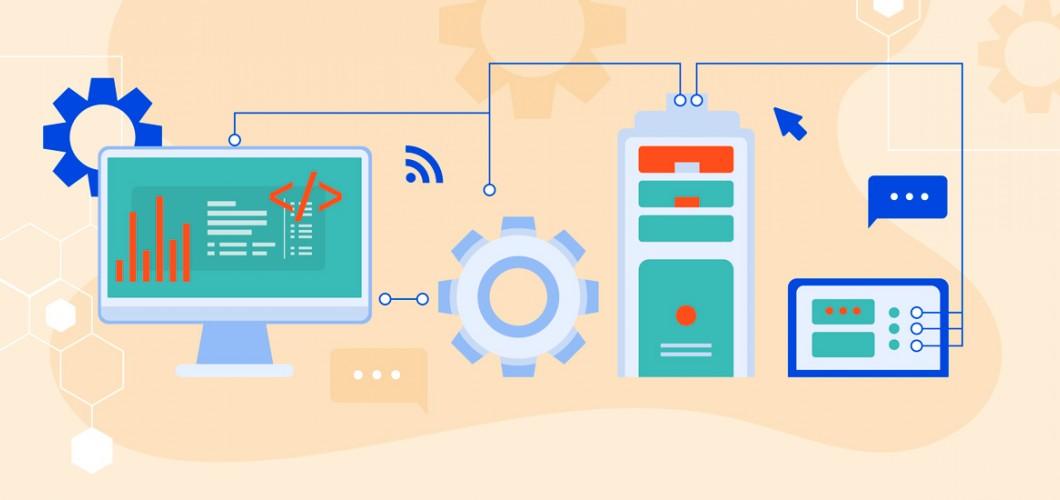
API Security and JWT Usage in JavaScript: A Comprehensive Guide
API security plays a crucial role in modern web applications. Today, applications exchange data between clients and servers, and security should always be a priority. JSON Web Token (JWT) is a widely used method for securing APIs and managing user authentication processes. In this article, we will explore how to use JWT for API security with JavaScript, providing detailed examples and explanations.
1. Why is API Security Important?
APIs (Application Programming Interfaces) enable communication between different software components. Web and mobile applications send and receive data through APIs. However, security vulnerabilities may arise during this data exchange.
API security ensures that data is protected from malicious actors, unauthorized access is blocked, and sensitive information like user details is safeguarded. It includes aspects like encryption, authentication, authorization, and more.
Why is API security important?
- Data Security: Prevents data from being stolen or tampered with.
- Authentication: Ensures that only authorized users can access the system.
- Authorization: Restricts users to only the data they are permitted to access.
- Data Integrity: Ensures that data is transmitted correctly without alteration.
2. What is JSON Web Token (JWT)?
JSON Web Token (JWT) is a standard used primarily for user authentication and authorization in APIs. JWT is a digital authentication token that is transmitted between the server and client to verify a user's identity. A JWT consists of three main components: Header, Payload, and Signature.
JWT Structure:
- Header: Specifies the algorithm used (e.g., HMAC SHA256 or RSA).
- Payload: Contains the data, such as authentication information. This data is usually encoded in base64.
- Signature: Signs the header and payload. The signature ensures that the token is valid and hasn't been tampered with.
Advantages of JWT:
- Portability: JWT can be carried across any device. Instead of sending user data to servers each time, authentication is done with the JWT.
- Stateless: JWT doesn't require the server to store session information. All user information is carried within the token.
- Security: JWT is signed, meaning it cannot be altered. Also, when sent over HTTPS, it can be transmitted securely.
3. How JWT Works
The principle behind JWT is simple:
- User Login: The user logs into the system with a username and password.
- JWT Creation: Upon successful login, the server generates a JWT and sends it to the client.
- API Requests: The client sends this token in the Authorization header with each API request.
- Token Verification: The server verifies the token. If the token is valid, the API request is processed; if invalid, access is denied.
4. API Security with JWT
Now, let's explain how to secure APIs using JWT with JavaScript in detail.
Step 1: Create JWT and User Authentication
After the user logs in, the server generates a JWT for them. Here's an example of how to do this:
Backend - Node.js and Express.js JWT Creation
const express = require('express');
const jwt = require('jsonwebtoken');
const app = express();
const bodyParser = require('body-parser');
app.use(bodyParser.json());
// User authentication process
app.post('/login', (req, res) => {
const { username, password } = req.body;
// Simple validation (in real applications, database check is done)
if (username === 'admin' && password === 'password') {
// Create JWT
const token = jwt.sign({ username: 'admin' }, 'secretkey', { expiresIn: '1h' });
res.json({ token });
} else {
res.status(401).send('Authentication failed');
}
});
// Start server
app.listen(3000, () => {
console.log('Server running on port 3000');
});
In this example, after the user logs in, the server generates a JWT and sends it to the client. This JWT is a token that the server has digitally signed and authenticated.
Step 2: Sending JWT with API Requests
The client sends this JWT with each API request under the Authorization header.
Frontend - Sending JWT with JavaScript
const token = localStorage.getItem('token'); // Get JWT
fetch('http://localhost:3000/protected', {
method: 'GET',
headers: {
'Authorization': `Bearer ${token}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Step 3: JWT Verification
The server verifies the incoming JWT to authenticate the API request:
Backend - JWT Verification
const authenticateToken = (req, res, next) => {
const token = req.header('Authorization')?.split(' ')[1];
if (!token) {
return res.status(403).send('Token required');
}
jwt.verify(token, 'secretkey', (err, user) => {
if (err) {
return res.status(403).send('Invalid token');
}
req.user = user;
next();
});
};
app.get('/protected', authenticateToken, (req, res) => {
res.json({ message: 'Protected data', user: req.user });
});
In this code, the server verifies the token in each request. If the token is valid, protected data is sent to the client.
5. JWT Security Tips
Here are some important security tips when using JWT:
- Use Strong Keys: The key used for signing JWT should be strong and kept secret.
- Use HTTPS: Always send JWT over HTTPS. Otherwise, the token can be intercepted by malicious parties.
- Token Expiration: JWT tokens should have an expiration time. Expired tokens should be rejected.
- Use Refresh Tokens: For long sessions, use Refresh Tokens to obtain a new JWT when the main token expires.
Conclusion
JWT is a powerful tool for securing APIs with JavaScript. JSON Web Token simplifies user authentication and authorization processes while making your APIs more secure. In this guide, we learned how JWT works, how to create and verify tokens, and how to secure APIs using JWT.
As API security becomes increasingly critical, using methods like JWT to secure your applications is a vital step toward building a secure environment for your users.
Leave a Comment