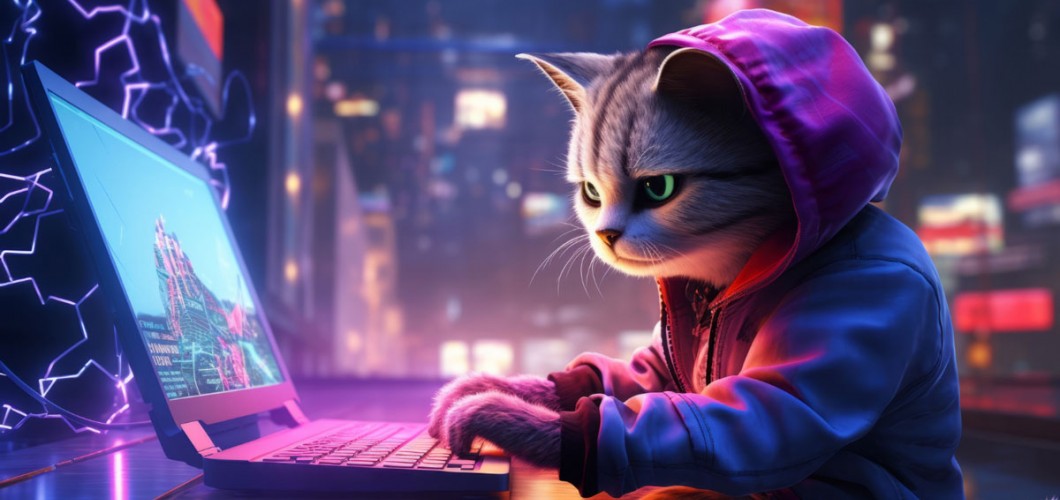
Building Real-Time Applications with WebSockets in JavaScript
Introduction
Modern web applications increasingly require instant and bidirectional communication between the client and server. WebSockets are one of the most effective solutions for meeting this need.
In this article, we'll explore how to use WebSockets in JavaScript, cover core concepts, provide detailed examples, and discuss how to build real-time applications.
What is WebSockets?
WebSockets is a communication protocol that enables continuous, bidirectional, and low-latency communication between the client and server. Unlike HTTP, where a new connection is established for each request, WebSockets maintain a persistent connection, enabling faster and more efficient data exchange.
Key Features of WebSockets
- Bidirectional Communication: Both the client and server can send data.
- Low Latency: Enables real-time data exchange.
- Persistent Connection: Once established, the connection remains open.
- Ideal for Real-Time Use Cases: Perfect for chat apps, live notifications, and multiplayer games.
How Do WebSockets Work?
- Handshake: The client initiates an HTTP request to the server and specifies the WebSocket protocol.
- Connection Setup: The server acknowledges and upgrades the connection to WebSocket.
- Data Transfer: Both sides can now send messages to each other.
- Connection Closure: Either side can terminate the connection.
Building a Simple WebSocket Application
1. Setting Up a Node.js WebSocket Server
First, install the ws
package:
npm install ws
server.js
const WebSocket = require('ws');
// Creating the server
const server = new WebSocket.Server({ port: 8080 });
server.on('connection', (socket) => {
console.log('A new user has connected.');
// Listening for messages
socket.on('message', (message) => {
console.log(`Received Message: ${message}`);
// Broadcasting the message to all connected clients
server.clients.forEach(client => {
if (client.readyState === WebSocket.OPEN) {
client.send(`Server: ${message}`);
}
});
});
// On connection close
socket.on('close', () => {
console.log('A user has disconnected.');
});
// Initial message from server to client
socket.send('Connection established!');
});
2. Creating a Basic HTML Client
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>WebSocket Chat</title>
</head>
<body>
<h2>WebSocket Chat Application</h2>
<input type="text" id="message" placeholder="Type your message..." />
<button onclick="sendMessage()">Send</button>
<ul id="chat"></ul>
<script>
const socket = new WebSocket('ws://localhost:8080');
const chat = document.getElementById('chat');
// Handling incoming messages
socket.onmessage = function(event) {
const li = document.createElement('li');
li.textContent = event.data;
chat.appendChild(li);
};
function sendMessage() {
const message = document.getElementById('message').value;
socket.send(message);
}
</script>
</body>
</html>
How WebSocket Communication Works
- Connection Setup: The client connects to the server using
new WebSocket('ws://localhost:8080')
. - Sending Messages: Messages are sent with
socket.send()
. - Receiving Messages: The client listens for incoming messages using
socket.onmessage
.
Advantages of Using WebSockets
- ???? Real-Time Communication: Faster and more immediate than HTTP.
- ⚡ Low Latency: Minimal delay due to the persistent connection.
- ???? Bidirectional Communication: Both server and client can send data.
- ???? Reduced Network Traffic: Only one persistent connection is maintained.
Common Use Cases for WebSockets
- Chat Applications: Prevents delays in message delivery.
- Live Notification Systems: Instant notifications for emails, news, and more.
- Online Games: Real-time updates in multiplayer games.
- Financial Dashboards: For continuous data streaming and updates.
Managing WebSocket Connections
Closing a Connection:
socket.close();
Handling Connection Errors:
socket.onerror = function(error) {
console.error('WebSocket Error:', error);
};
Checking Connection Status:
if (socket.readyState === WebSocket.OPEN) {
console.log('Connection is open');
}
WebSocket Security Tips
- Use WSS Protocol: Always use
wss://
for secure communication. - Authentication: Implement token-based authentication during the handshake.
- Monitor Connection Status: Regularly check if the connection is alive.
- Validate Incoming Data: Always sanitize and validate messages from clients.
Conclusion
WebSockets offer one of the most effective ways to build real-time applications with JavaScript. This protocol allows seamless, fast, and bidirectional communication between clients and servers.
By leveraging WebSockets, especially for chat applications, live notification systems, or online games, you can significantly enhance both performance and user experience.
Leave a Comment