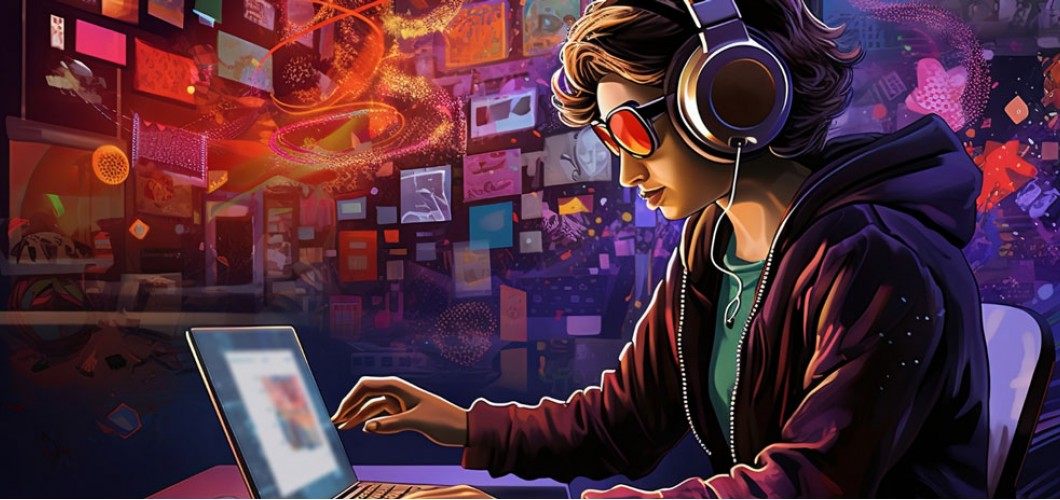
Here's a comprehensive and detailed blog post on "JavaScript and GraphQL: Basic and Advanced Usage" with examples, visuals, and explanations. ????
JavaScript and GraphQL: Basic and Advanced Usage
Introduction
In modern web development, managing data effectively between the client and server is crucial. While REST APIs have been a standard, GraphQL is revolutionizing the way data is fetched and manipulated, offering more efficiency and flexibility.
In this guide, you'll learn the fundamentals and advanced concepts of using GraphQL with JavaScript. We'll cover everything from setup to advanced query optimizations, complete with examples.
???? What is GraphQL?
GraphQL is an open-source query language for APIs, developed by Facebook. It enables clients to request exactly the data they need, reducing over-fetching and under-fetching.
Key Features of GraphQL
- ???? Declarative Data Fetching: Fetch exactly the data you need in a single request.
- ⚡ Efficient: Reduces network usage by avoiding unnecessary data transfer.
- ???? Strongly Typed Schema: Ensures data consistency and clarity.
- ???? Real-Time Updates: Supports real-time data through subscriptions.
???? Setting Up a Basic GraphQL Server with Node.js
First, install the necessary packages:
npm init -y
npm install express express-graphql graphql
server.js
const express = require('express');
const { graphqlHTTP } = require('express-graphql');
const { buildSchema } = require('graphql');
// Define the schema
const schema = buildSchema(`
type Query {
hello: String
greet(name: String!): String
}
`);
// Define the root resolver
const root = {
hello: () => 'Hello, World!',
greet: ({ name }) => `Hello, ${name}!`,
};
// Create the Express server
const app = express();
app.use('/graphql', graphqlHTTP({
schema: schema,
rootValue: root,
graphiql: true, // Enable GraphiQL GUI
}));
app.listen(4000, () => console.log('Server is running on http://localhost:4000/graphql'));
Running the Server
node server.js
Visit http://localhost:4000/graphql
to test queries using the built-in GraphiQL interface.
???? Basic GraphQL Queries
1. Simple Query
{
hello
}
Response:
{
"data": {
"hello": "Hello, World!"
}
}
2. Query with Variables
{
greet(name: "Alice")
}
Response:
{
"data": {
"greet": "Hello, Alice!"
}
}
????️ Advanced GraphQL Concepts
1. Defining Custom Types and Resolvers
Let's build a user system with custom types.
const schema = buildSchema(`
type User {
id: ID!
name: String!
email: String!
}
type Query {
getUser(id: ID!): User
}
type Mutation {
createUser(name: String!, email: String!): User
}
`);
const users = [];
let idCounter = 1;
const root = {
getUser: ({ id }) => users.find(user => user.id === id),
createUser: ({ name, email }) => {
const user = { id: idCounter++, name, email };
users.push(user);
return user;
},
};
2. Performing Mutations
Create a User:
mutation {
createUser(name: "John Doe", email: "john@example.com") {
id
name
email
}
}
Response:
{
"data": {
"createUser": {
"id": "1",
"name": "John Doe",
"email": "john@example.com"
}
}
}
3. Using Variables in Queries
query getUser($userId: ID!) {
getUser(id: $userId) {
id
name
email
}
}
Variables:
{
"userId": "1"
}
⚡ GraphQL with Real-Time Data (Subscriptions)
GraphQL can handle real-time updates using subscriptions with WebSockets.
Installation:
npm install graphql-subscriptions ws
Setting Up a Basic Subscription
Due to complexity, subscriptions typically require libraries like Apollo Server. However, here's a conceptual approach:
- Setup Subscription Schema
- Trigger Events
- Listen to Events on the Client
✅ Best Practices for GraphQL Development
- Use Strongly Typed Schemas: It enhances data integrity and error handling.
- Implement Pagination and Filtering: Avoid heavy data loads.
- Batch Queries: Prevent N+1 problems with libraries like DataLoader.
- Secure the API: Use authentication and authorization checks.
- Optimize Performance: Minimize resolver overhead and use efficient data sources.
???? REST vs. GraphQL
Feature | REST | GraphQL |
---|---|---|
Data Fetching | Multiple Endpoints | Single Endpoint |
Data Over-fetching | Yes | No |
Real-Time Support | Limited | Native Support (Subscriptions) |
Flexibility | Less Flexible | Highly Flexible |
???? GraphQL Clients: Using Apollo Client in JavaScript
Install Apollo Client:
npm install @apollo/client graphql
Client Setup (React Example)
import { ApolloClient, InMemoryCache, ApolloProvider, gql, useQuery } from '@apollo/client';
const client = new ApolloClient({
uri: 'http://localhost:4000/graphql',
cache: new InMemoryCache()
});
function App() {
return (
<ApolloProvider client={client}>
<Users />
</ApolloProvider>
);
}
function Users() {
const { loading, error, data } = useQuery(gql`
{
getUser(id: "1") {
name
email
}
}
`);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error :(</p>;
return <div>{data.getUser.name}</div>;
}
???? Conclusion
GraphQL is a game-changer for modern API development. By combining it with JavaScript, developers can:
- Build efficient and scalable APIs
- Enhance flexibility in data fetching
- Reduce network overhead
- Support real-time communication
Whether you're a beginner or diving into advanced concepts like subscriptions, GraphQL offers the tools needed for powerful and efficient API development.
If you'd like to expand this guide further or need more examples, feel free to ask! ????
Leave a Comment