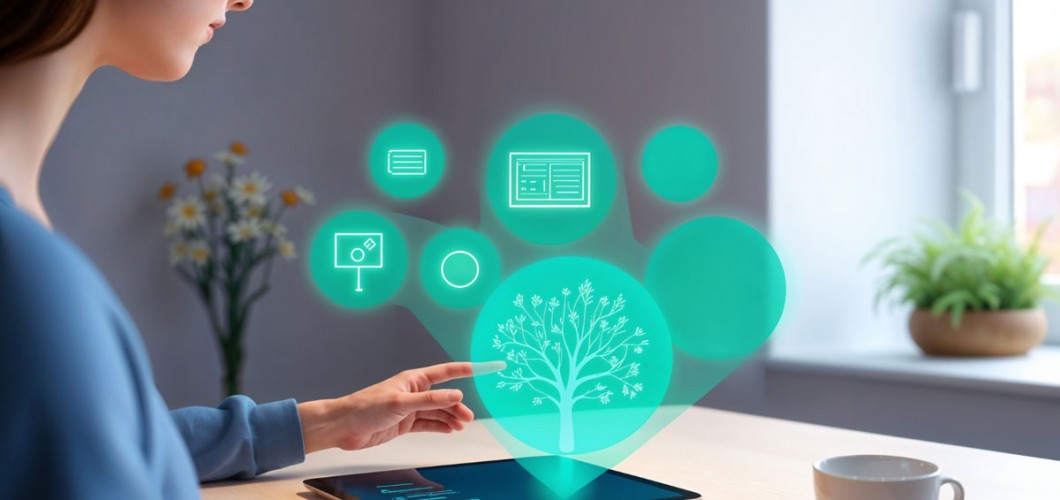
JavaScript and IoT (Internet of Things) Projects: Key Concepts and Applications
The Internet of Things (IoT) is a concept that enables devices to communicate with each other over the internet. IoT projects can be applied in a wide range of fields, from smart homes to industrial devices. While JavaScript is primarily used for web applications, it can also be effectively used in IoT projects. In this blog post, we will explore the process of developing IoT projects using JavaScript, providing examples and detailed explanations along the way.
1. What is IoT and Why JavaScript?
1.1. What is IoT?
The Internet of Things (IoT) refers to the connection and communication of physical devices over the internet. These devices can include sensors, gadgets, and other smart systems. IoT projects gather data from various sources, process it, and provide insights through analysis.
1.2. JavaScript and IoT
While JavaScript is typically used for browser-based applications, it is also well-suited for IoT projects. With the help of Node.js, JavaScript can also be run on the server side to manage communication between devices. JavaScript simplifies tasks such as collecting data from devices, processing it, and developing user interfaces.
2. Advantages of Using JavaScript in IoT Projects
2.1. Easy to Learn
JavaScript is an easy-to-learn language, especially for beginners. This makes it easier for newcomers to start building IoT projects quickly.
2.2. Node.js and Backend Applications
Node.js is a platform that allows JavaScript to run on the server side. This platform is perfect for building backend applications that interact with IoT devices. Node.js is ideal for creating fast, low-latency, and scalable applications.
2.3. Web-Based Interfaces
JavaScript is the ideal language for building web-based user interfaces (UIs). The applications you develop for managing IoT devices can feature sleek, interactive UIs that allow users to control devices and visualize data.
3. Developing IoT Projects with JavaScript: Step-by-Step Guide
3.1. Step 1: Installing the Necessary Tools
To start using JavaScript in IoT projects, you will need a few tools:
- Node.js: This is required to run JavaScript on the server side.
- MQTT Protocol: This lightweight messaging protocol is ideal for communication between IoT devices.
- Johnny-Five: A JavaScript library for interacting with microcontrollers such as Arduino.
# Install Node.js with the following command:
sudo apt install nodejs
3.2. Step 2: Creating a Simple IoT Device with Johnny-Five
Johnny-Five makes it easy to develop IoT projects with microcontrollers like Arduino. Below is an example to control a simple LED.
Materials Needed:
- Arduino board
- LED
- Resistor
Control an LED with Node.js:
- Install the Johnny-Five library:
npm install johnny-five
- Use the following JavaScript code to control the LED on the Arduino board:
const five = require("johnny-five");
const board = new five.Board();
board.on("ready", function() {
const led = new five.Led(13); // LED pin number
led.blink(500); // Blink LED every 500ms
});
This simple application will make the LED on your Arduino board blink. Johnny-Five allows you to easily access and control the input/output pins on your devices.
3.3. Step 3: Communication Between IoT Devices with MQTT
MQTT (Message Queuing Telemetry Transport) is a lightweight protocol for communication between IoT devices. You can use MQTT with JavaScript to exchange messages between devices.
First, install the MQTT library:
npm install mqtt
Here’s an example of an MQTT client to send and receive messages:
const mqtt = require('mqtt');
const client = mqtt.connect('mqtt://test.mosquitto.org');
client.on('connect', function () {
console.log('Connected to MQTT broker');
client.subscribe('iot/topic', function (err) {
if (!err) {
client.publish('iot/topic', 'Hello from JavaScript IoT!');
}
});
});
client.on('message', function (topic, message) {
console.log(message.toString());
});
This basic MQTT client sends a message and then receives the same message. This type of communication allows IoT devices to interact with each other.
4. Using JavaScript and Web Applications for IoT Projects
IoT devices typically gather data and send it to a server. Web applications can then visualize this data. You can use JavaScript and web technologies (HTML, CSS, React, Vue, etc.) to visualize IoT data.
Visualizing IoT Data with React:
You can display IoT data in a web application using React. Here’s a simple example:
import React, { useState, useEffect } from 'react';
function IoTData() {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data from the API
fetch('http://iotdevice.com/api/data')
.then(response => response.json())
.then(data => setData(data));
}, []);
if (!data) return <div>Loading...</div>;
return (
<div>
<h1>IoT Device Data</h1>
<p>Temperature: {data.temperature}°C</p>
<p>Humidity: {data.humidity}%</p>
</div>
);
}
export default IoTData;
In this example, data from an IoT device is visualized in a web application.
5. The Future of IoT Projects and JavaScript
The future of IoT projects is shaped by the increasing number of devices that are connected to the internet and exchange data. JavaScript offers great potential for IoT projects. You can use JavaScript and Node.js to interact with low-level devices and create user-friendly web interfaces.
6. Conclusion
JavaScript is a powerful tool for IoT projects. With technologies like Node.js and MQTT, you can easily communicate between devices and process data. You can also visualize and control IoT devices through web-based applications. JavaScript provides vast opportunities for creating innovative IoT projects.
Leave a Comment