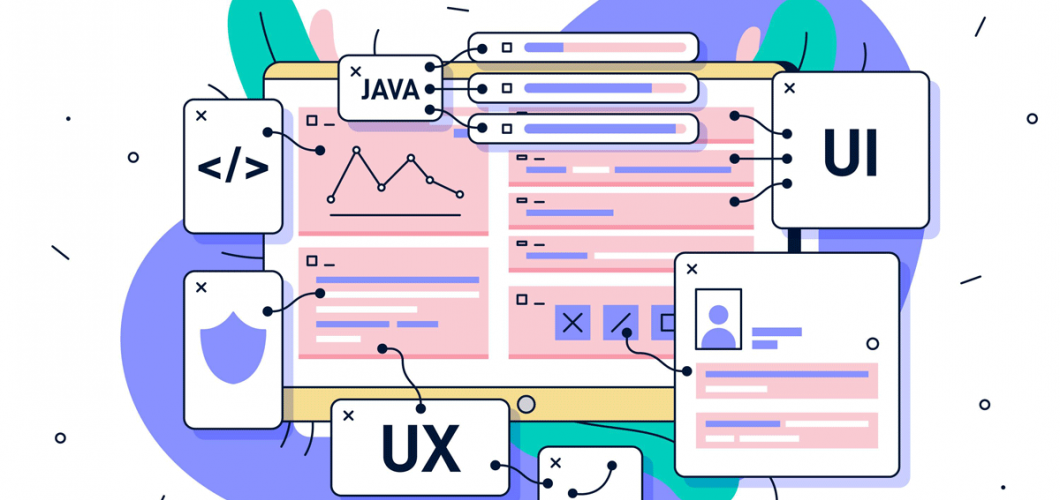
JavaScript Date and Time Operations: The Date Object
JavaScript allows us to easily perform operations involving date and time, which are frequently used in web applications. For example, displaying the date to the user, adding timestamps, or comparing dates. One of the main tools used for date and time operations in JavaScript is the Date object. In this post, we will provide a comprehensive guide on how to use the Date object in JavaScript for date and time operations.
What is the Date Object?
The Date
object in JavaScript represents a specific point in time (date and time). It is used to manipulate, compare, and format dates and times. The Date
object provides a variety of methods for getting and setting date and time information.
In JavaScript, using the Date
object, you can:
- Get the current date and time
- Compare dates
- Format dates
- Perform date arithmetic (add, subtract, etc.)
Getting the Current Date and Time
By default, when you create a new Date
object, it automatically gets the current date and time. If you don’t pass any parameters to the Date
constructor, it will give you the current date and time.
Example:
const currentDate = new Date();
console.log(currentDate); // Example output: Mon Mar 14 2025 16:43:19 GMT+0300 (GMT+03:00)
In this example, new Date()
returns the current date and time. The date and time will be displayed in the local timezone of your environment.
Creating a Specific Date with the Date Object
You can also create specific dates by passing parameters to the Date
constructor. Dates can be provided in various formats.
Example 1: Year, Month, Day
const specificDate = new Date(2025, 2, 14); // Months are 0-based (0 = January, 1 = February, etc.)
console.log(specificDate); // Fri Mar 14 2025 00:00:00 GMT+0300 (GMT+03:00)
In this example, new Date(2025, 2, 14)
creates the date March 14, 2025. Note that months in JavaScript start from 0, so 2
represents March.
Example 2: Year, Month, Day, Hour, Minute, Second, Millisecond
const specificDateTime = new Date(2025, 2, 14, 10, 30, 0, 0); // March 14, 2025, 10:30:00
console.log(specificDateTime); // Fri Mar 14 2025 10:30:00 GMT+0300 (GMT+03:00)
In this case, we create a specific date and time (March 14, 2025, at 10:30 AM).
Accessing Date Information with the Date Object
Once you have a Date
object, you can use various methods to access the individual components of the date and time.
Example: Accessing Date and Time Components
const currentDate = new Date();
console.log("Year:", currentDate.getFullYear()); // Year
console.log("Month:", currentDate.getMonth()); // Month (0-11 range)
console.log("Day:", currentDate.getDate()); // Day (1-31 range)
console.log("Hour:", currentDate.getHours()); // Hour (0-23 range)
console.log("Minute:", currentDate.getMinutes()); // Minute (0-59 range)
console.log("Second:", currentDate.getSeconds()); // Second (0-59 range)
console.log("Millisecond:", currentDate.getMilliseconds()); // Millisecond (0-999 range)
This code will break down the current date and time into components (year, month, day, hour, etc.) and log them to the console.
Manipulating Dates with the Date Object
You can manipulate dates using various methods provided by the Date
object. For example, you can add days, months, or years to a date.
Example: Adding One Day
To add a day to a Date
object, you can use the setDate()
method. Here’s how to add one day to the current date:
const currentDate = new Date();
console.log("Today:", currentDate);
currentDate.setDate(currentDate.getDate() + 1); // Add one day
console.log("Tomorrow:", currentDate);
In this example, the setDate()
method adds one day to the current date.
Example: Adding One Month
To add a month to a Date
object, you can use the setMonth()
method:
const currentDate = new Date();
console.log("Today:", currentDate);
currentDate.setMonth(currentDate.getMonth() + 1); // Add one month
console.log("One Month Later:", currentDate);
In this example, the setMonth()
method adds one month to the current date.
Example: Adding One Year
To add a year to a Date
object, you can use the setFullYear()
method:
const currentDate = new Date();
console.log("Today:", currentDate);
currentDate.setFullYear(currentDate.getFullYear() + 1); // Add one year
console.log("One Year Later:", currentDate);
Formatting Dates with the Date Object
JavaScript provides several methods for formatting dates, such as toLocaleDateString()
, toLocaleTimeString()
, and toLocaleString()
for specific formats.
Example: Formatting a Date
const currentDate = new Date();
const formattedDate = currentDate.toLocaleDateString('en-US'); // US format
console.log("Date:", formattedDate); // 03/14/2025
Example: Formatting Date and Time
const currentDate = new Date();
const formattedDateTime = currentDate.toLocaleString('en-US'); // US format
console.log("Date and Time:", formattedDateTime); // 3/14/2025, 4:43:19 PM
Calculating Time Differences Between Two Dates
You can calculate the difference between two dates using the getTime()
method, which returns the time in milliseconds.
Example: Difference Between Two Dates
const date1 = new Date('2025-03-14T10:00:00');
const date2 = new Date('2025-03-14T12:00:00');
const timeDifference = date2.getTime() - date1.getTime();
const hoursDifference = timeDifference / (1000 * 3600); // Milliseconds to hours
console.log("Hour Difference:", hoursDifference); // 2
In this example, we calculate the difference between two dates in hours.
Conclusion
The Date object in JavaScript is a powerful tool for working with dates and times. With it, you can get the current date and time, compare dates, perform date arithmetic, and format dates for display. By mastering the Date
object, you can manipulate date and time data efficiently in your web applications.
Date and time operations are commonly needed in web development, and understanding how to use the Date
object effectively can make your code more robust and flexible. We hope this guide helps you to better understand JavaScript's capabilities for working with dates and times.
Leave a Comment