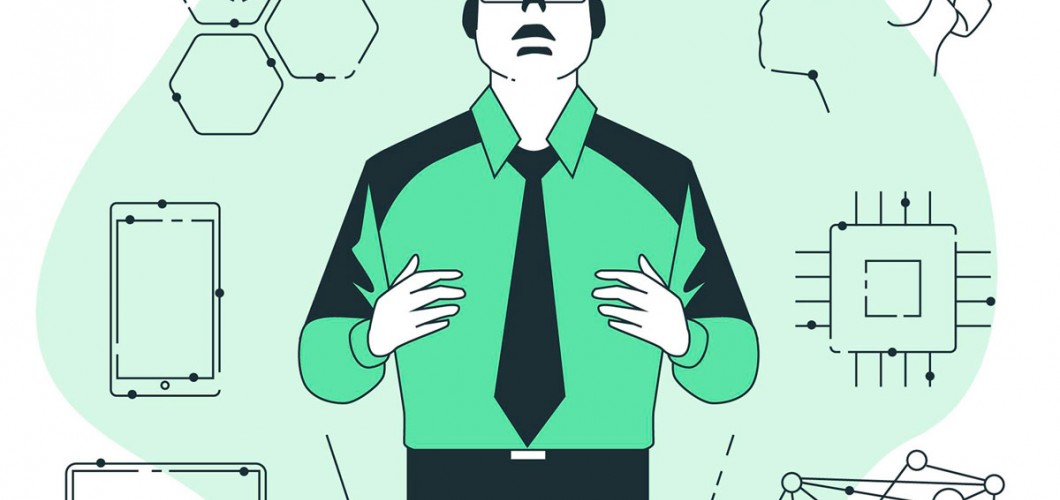
JavaScript Modules: import, export, and Modular Programming
JavaScript modules enable developers to break code into smaller, manageable, and reusable pieces, enhancing maintainability and scalability. In this comprehensive guide, we will explore how modules work in JavaScript, focusing on the import
and export
syntax and best practices for modular programming.
1. What is a JavaScript Module?
A JavaScript module is a file that contains code (variables, functions, classes) that can be reused in other files. Modules help organize code, avoid naming conflicts, and make it easier to maintain.
2. Exporting in JavaScript
To make functions, variables, or classes accessible to other modules, you need to export them.
Named Exports
Named exports allow you to export multiple items from a module.
math.js
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
You can also export items in a single statement:
const multiply = (a, b) => a * b;
const divide = (a, b) => a / b;
export { multiply, divide };
Default Export
A module can have only one default export.
logger.js
export default function log(message) {
console.log(message);
}
3. Importing in JavaScript
To use exported items from another module, use the import
statement.
Importing Named Exports
import { add, subtract } from './math.js';
console.log(add(2, 3)); // 5
console.log(subtract(5, 2)); // 3
You can also rename imports using as
:
import { add as addition } from './math.js';
console.log(addition(2, 3)); // 5
Importing Default Exports
import log from './logger.js';
log('This is a default export');
Importing Everything
import * as math from './math.js';
console.log(math.add(1, 2)); // 3
console.log(math.subtract(5, 2)); // 3
4. Dynamic Imports
Dynamic imports allow you to load modules on demand.
async function loadModule() {
const module = await import('./math.js');
console.log(module.add(2, 3));
}
loadModule();
5. Benefits of Modular Programming
- Maintainability: Smaller modules are easier to debug and maintain.
- Reusability: Modules can be reused across projects.
- Scalability: Modular code is easier to scale.
- Namespace Management: Reduces naming conflicts by encapsulating code.
6. Best Practices for Modular Programming
- One Module, One Responsibility: Each module should focus on a specific functionality.
- Avoid Circular Dependencies: Ensure modules don’t depend on each other in a circular manner.
- Use Default Exports for Single Entities: When exporting a single item, prefer default exports.
- Consistent Naming Conventions: Use clear and consistent names for exports.
Conclusion
Understanding how to work with modules in JavaScript is fundamental for writing clean and efficient code. By leveraging import
and export
syntax and following modular programming practices, you can build scalable and maintainable JavaScript applications.
Feel free to ask questions or share your thoughts in the comments!
Leave a Comment