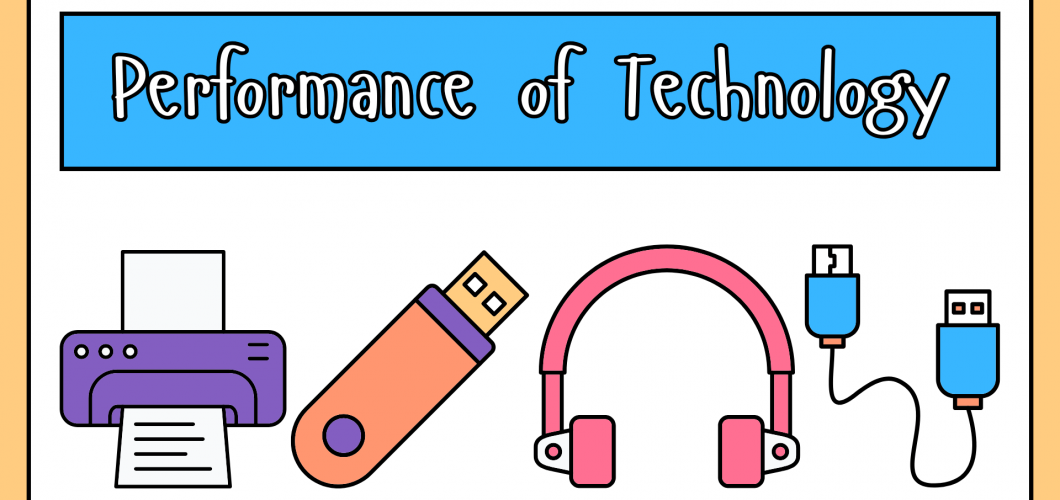
JavaScript Performance Optimization Techniques: A Comprehensive Guide
Introduction
Ensuring web applications run fast and efficiently is crucial for user experience. Optimizing JavaScript code helps reduce page load times, decrease resource usage, and provide a smoother experience. In this article, we will explore the most effective techniques for improving JavaScript performance in detail.
1. Code Optimization
1.1 Using Loops Efficiently
Loops impact performance significantly when handling large data sets. Optimizing loops can prevent unnecessary computations.
Good Example:
let array = [1, 2, 3, 4, 5];
for (let i = 0, len = array.length; i < len; i++) {
console.log(array[i]);
}
Bad Example:
let array = [1, 2, 3, 4, 5];
for (let i = 0; i < array.length; i++) {
console.log(array[i]);
}
The bad example recalculates array.length
in each iteration, causing unnecessary performance loss.
1.2 Using forEach
, map
, and reduce
These methods enhance readability and can improve performance.
let numbers = [1, 2, 3, 4, 5];
numbers.forEach(num => console.log(num));
Using forEach
instead of a for
loop can be beneficial in some cases. Always test for optimal performance.
2. Memory Optimization
2.1 Cleaning Up Unused Variables and Objects
Avoid unnecessary variable and object usage.
let obj = {name: "Ali", age: 25};
obj = null; // Free up memory
While JavaScript's garbage collector removes unused variables, manually freeing large objects improves performance.
2.2 Using const
and let
Replacing var
with const
and let
results in better memory management.
const PI = 3.14; // Use `const` for immutable values
let counter = 0; // Use `let` for mutable values
3. DOM Optimization
3.1 Minimizing DOM Manipulations
DOM operations are expensive and should be minimized.
Bad Usage:
for (let i = 0; i < 100; i++) {
let div = document.createElement("div");
div.textContent = "Element " + i;
document.body.appendChild(div);
}
Good Usage:
let fragment = document.createDocumentFragment();
for (let i = 0; i < 100; i++) {
let div = document.createElement("div");
div.textContent = "Element " + i;
fragment.appendChild(div);
}
document.body.appendChild(fragment);
This method improves performance by reducing direct DOM updates.
4. Asynchronous Programming
4.1 Using async
and await
Prevent blocking operations using async
and await
.
async function fetchData() {
let response = await fetch("https://api.example.com/data");
let data = await response.json();
console.log(data);
}
This ensures the main thread continues running without being blocked.
5. Network Performance Optimization
5.1 Reducing Unnecessary HTTP Requests
Minimize HTTP requests by using batch requests.
Promise.all([
fetch("https://api.example.com/user"),
fetch("https://api.example.com/orders")
]).then(responses => Promise.all(responses.map(res => res.json())))
.then(data => console.log(data));
5.2 Using Lazy Loading
Deferring large assets until needed reduces initial load time.
<img src="placeholder.jpg" data-src="real-image.jpg" class="lazy-load">
Lazy load implementation in JavaScript:
document.addEventListener("DOMContentLoaded", function() {
let lazyImages = document.querySelectorAll(".lazy-load");
lazyImages.forEach(img => img.src = img.dataset.src);
});
6. Browser Optimization
6.1 Using Web Workers
Offload heavy tasks to web workers to prevent UI freezing.
let worker = new Worker("worker.js");
worker.postMessage("start");
worker.onmessage = function(e) {
console.log("Result: ", e.data);
};
This method improves performance by running tasks in the background.
Conclusion
JavaScript performance optimization is essential for enhancing speed and efficiency. Efficient loop usage, proper memory management, reduced DOM manipulations, and asynchronous programming can significantly boost performance. Start applying these techniques for faster and more efficient applications!
Leave a Comment