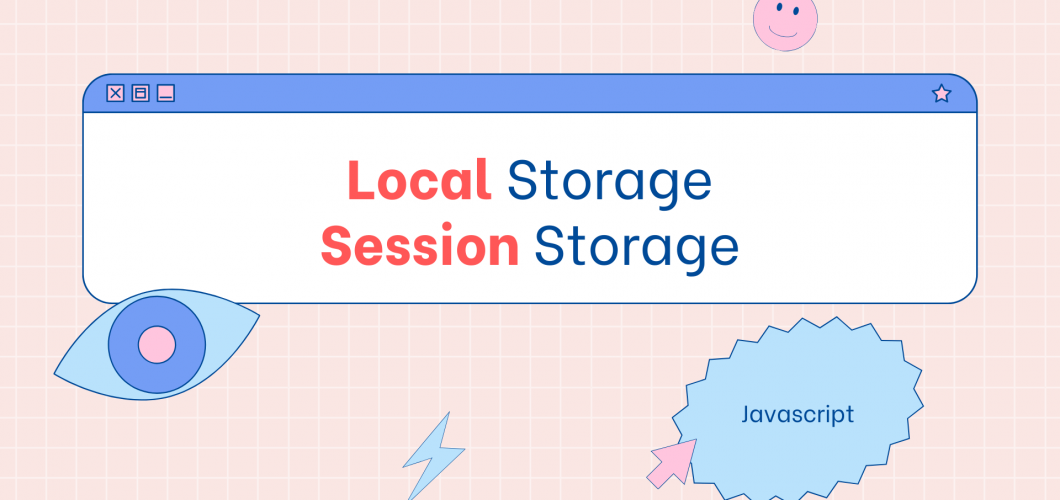
Using Web Storage API in JavaScript (localStorage, sessionStorage)
Introduction
In the world of web development, storing user data in the browser plays a crucial role for modern applications. The Web Storage API provides a simple and effective way to store data in the browser. This API allows you to store data on the user's browser, preventing data loss between page refreshes or session terminations.
The Web Storage API consists of two main components: localStorage and sessionStorage. Both allow you to store key-value pairs, but the difference between them lies in how long the data is stored.
In this article, we will explore what the Web Storage API is, how it works, and the differences between localStorage and sessionStorage in detail. We will also provide examples of using both APIs.
What is Web Storage API?
The Web Storage API is a way to store data in the browser. It provides two main storage options: localStorage and sessionStorage. This API allows easy interaction with JavaScript and enables storing, retrieving, and deleting data.
Both storage options store data as key-value pairs, which makes it easy to manage the data directly via JavaScript.
Advantages of Web Storage API:
- Data Persistence: Data is stored in the browser and does not get lost between page refreshes.
- Ease of Use: Data is stored as key-value pairs, making it easy to access.
- Fast Access: You can quickly access the stored data.
Differences Between localStorage and sessionStorage
localStorage
- Data Duration: Data is persistent, meaning it does not get lost when the browser is closed and reopened.
- Storage Capacity: Typically provides between 5-10 MB of storage space.
- Access: Accessible across page reloads or even after closing and reopening the browser.
sessionStorage
- Data Duration: Data is stored only for the duration of the session (current page). It is lost when the browser tab is closed.
- Storage Capacity: Typically provides between 5-10 MB of storage space.
- Access: Accessible only within the current session (page). It is not available across tabs or windows.
Using the Web Storage API
Storing, retrieving, and deleting data with the Web Storage API is quite simple. Here are the basic methods:
1. Using localStorage
localStorage allows you to store data persistently. Even if the user refreshes the page or reopens the browser, the data remains.
Storing Data
You can use the localStorage.setItem()
method to store data:
// Storing data
localStorage.setItem('username', 'JohnDoe');
Retrieving Data
Use the localStorage.getItem()
method to retrieve data:
// Retrieving data
let username = localStorage.getItem('username');
console.log(username); // Output: JohnDoe
Removing Data
Use the localStorage.removeItem()
method to remove data:
// Removing data
localStorage.removeItem('username');
Clearing All Data
Use the localStorage.clear()
method to clear all stored data:
// Clearing all data
localStorage.clear();
Example: Storing User Information
// Storing user information
localStorage.setItem('user', JSON.stringify({ name: 'John', age: 30 }));
// Retrieving data
let user = JSON.parse(localStorage.getItem('user'));
console.log(user.name); // John
console.log(user.age); // 30
2. Using sessionStorage
sessionStorage allows you to store data for the current session. The data is lost when the browser or tab is closed.
Storing Data
You can use the sessionStorage.setItem()
method to store data:
// Storing data
sessionStorage.setItem('sessionKey', 'sessionValue');
Retrieving Data
Use the sessionStorage.getItem()
method to retrieve data:
// Retrieving data
let sessionValue = sessionStorage.getItem('sessionKey');
console.log(sessionValue); // sessionValue
Removing Data
Use the sessionStorage.removeItem()
method to remove data:
// Removing data
sessionStorage.removeItem('sessionKey');
Clearing All Data
Use the sessionStorage.clear()
method to clear all stored data:
// Clearing all data
sessionStorage.clear();
Example: Storing Data Across Page Refresh
// Storing session data
sessionStorage.setItem('loginStatus', 'loggedIn');
// Retrieving data
let status = sessionStorage.getItem('loginStatus');
console.log(status); // loggedIn
Understanding the Differences Between localStorage and sessionStorage
Feature | localStorage | sessionStorage |
---|---|---|
Data Duration | Persistent (data remains even after closing the browser) | Temporary (data is lost when tab is closed) |
Storage Capacity | 5-10 MB | 5-10 MB |
Access | Accessible across pages and sessions | Only accessible within the current session |
Performance and Security
Since the Web Storage API stores data in the browser, it’s important to consider security as well. Especially, avoid storing sensitive data (such as passwords or authentication tokens) in localStorage or sessionStorage. For such data, consider using more secure methods (e.g., server-side session management).
Performance Tips:
- Avoid storing excessive data with Web Storage API.
- For large data (e.g., images or media files), use IndexedDB instead of Web Storage.
- If you need to frequently update data, store only the changed part rather than the entire data to improve performance.
Conclusion
The Web Storage API is a powerful and simple way to store data in the browser. You can effectively manage data with localStorage for persistent data storage and sessionStorage for temporary data.
Correctly storing your data can improve performance and enhance the user experience in modern web applications. However, avoid using Web Storage to store sensitive data for better security practices.
Leave a Comment