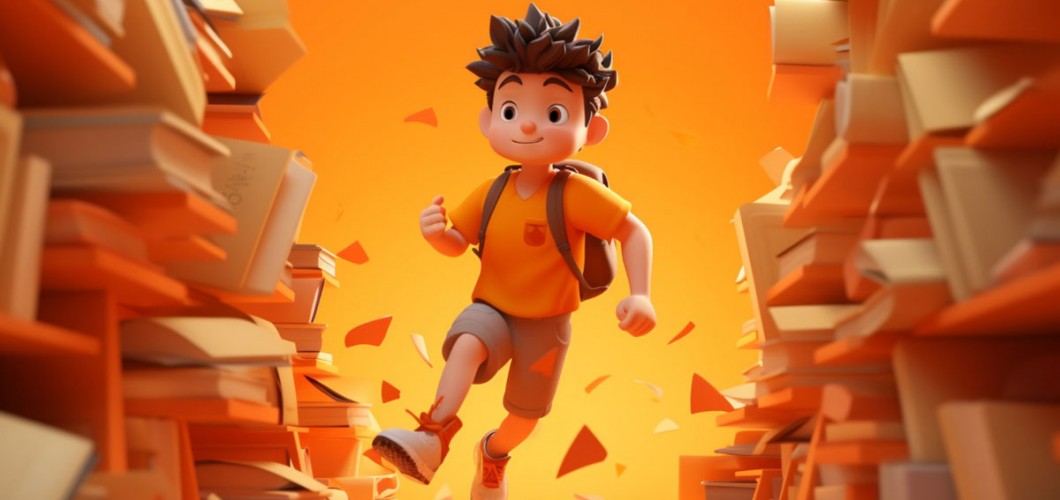
Vue.js Basic and Advanced Component Usage Guide
Vue.js has become an extremely popular JavaScript framework in recent years for web application development. Vue.js stands out for its component-based structure, reactive features, and ease of learning. In this article, we will cover both basic and advanced component usage in Vue.js, providing examples and explanations that will be useful for both beginners and advanced developers.
1. What Are Vue.js Components?
Vue.js components are isolated and reusable parts of an application that handle specific functionality. These components typically combine HTML, CSS, and JavaScript to provide functionality and presentation. Vue.js offers a system that makes managing components easy.
Basic Vue.js Component
To create a Vue component, you need to configure a Vue instance in a JavaScript file. Here's an example of a simple Vue component:
<template>
<div>
<h1>{{ message }}</h1>
</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello Vue.js!'
};
}
};
</script>
<style scoped>
h1 {
color: #42b983;
}
</style>
Explanations:
<template>
: Defines the HTML structure. This is where we define the visual content of the component.<script>
: Contains the JavaScript functionality for the component. Here, we define the component’s data, methods, and lifecycle functions.<style scoped>
: Styles specific to the component. Thescoped
attribute ensures that these styles are limited to this component.
Usage:
You can use Vue components within a Vue instance like this:
import Vue from 'vue';
import MyComponent from './MyComponent.vue';
new Vue({
el: '#app',
render: h => h(MyComponent)
});
2. Advanced Vue.js Component Usage
Vue.js offers many advanced features beyond basic component usage. In this section, we will explore component communication, dynamic components, component lifecycle, slots, and mixins, which are some of the advanced features Vue provides.
Component Communication
Vue.js offers several ways to pass data between components:
1. Props and Emit
- Props: Used to send data from a parent component to a child component.
- Emit: Used to send data from a child component to a parent component.
Example:
In the following example, the ParentComponent
sends data to the ChildComponent
:
ParentComponent.vue:
<template>
<div>
<ChildComponent :message="parentMessage" @updateMessage="updateMessage" />
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
parentMessage: 'Hello Child Component!'
};
},
methods: {
updateMessage(newMessage) {
this.parentMessage = newMessage;
}
}
};
</script>
ChildComponent.vue:
<template>
<div>
<h2>{{ message }}</h2>
<button @click="changeMessage">Change Message</button>
</div>
</template>
<script>
export default {
props: ['message'],
methods: {
changeMessage() {
this.$emit('updateMessage', 'New Message');
}
}
};
</script>
Dynamic Components
Vue.js allows you to manage dynamic components using the component
tag, enabling components to change dynamically based on certain conditions.
Example:
<template>
<div>
<component :is="currentComponent"></component>
<button @click="toggleComponent">Change Component</button>
</div>
</template>
<script>
import FirstComponent from './FirstComponent.vue';
import SecondComponent from './SecondComponent.vue';
export default {
data() {
return {
currentComponent: FirstComponent
};
},
methods: {
toggleComponent() {
this.currentComponent = this.currentComponent === FirstComponent ? SecondComponent : FirstComponent;
}
}
};
</script>
Component Lifecycle
The component lifecycle in Vue.js refers to the series of steps a component goes through from creation to destruction. Various lifecycle functions are triggered during this process. Here are the most commonly used lifecycle hooks:
created
: Triggered when the component is created.mounted
: Triggered when the component is mounted to the DOM.updated
: Triggered when the data changes.destroyed
: Triggered when the component is destroyed.
Example:
<template>
<div>
<h1>{{ count }}</h1>
<button @click="increment">Increment</button>
</div>
</template>
<script>
export default {
data() {
return {
count: 0
};
},
mounted() {
console.log('Component has been mounted to the DOM');
},
methods: {
increment() {
this.count++;
}
}
};
</script>
Slots: Passing Dynamic Content to Components
Vue.js slots allow you to pass dynamic content into a component, making the component more flexible.
Example:
<template>
<div>
<slot>Default Content</slot>
</div>
</template>
You can pass content to the slot like this:
<MyComponent>
<p>This content is passed to the slot</p>
</MyComponent>
Mixins: Reusing Functionality Across Components
Vue mixins allow you to reuse functionality across multiple components. Mixins let you add data, methods, and lifecycle hooks to components.
Example:
// myMixin.js
export const myMixin = {
data() {
return {
message: 'This is a mixin example'
};
},
methods: {
greet() {
console.log(this.message);
}
}
};
<template>
<div>
<button @click="greet">Greet</button>
</div>
</template>
<script>
import { myMixin } from './myMixin';
export default {
mixins: [myMixin]
};
</script>
3. Conclusion
By learning both basic and advanced component usage in Vue.js, we can take full advantage of Vue’s powerful features. Moving beyond basic component structures, we can create more flexible and scalable applications by utilizing features like component communication, dynamic components, lifecycle management, slots, and mixins.
The component-based architecture in Vue.js makes applications more modular and maintainable, while also allowing developers to manage their projects more efficiently. These features provide an excellent starting point for anyone looking to dive deeper into Vue.js. We hope this guide has inspired you to start building with Vue.js!
Leave a Comment