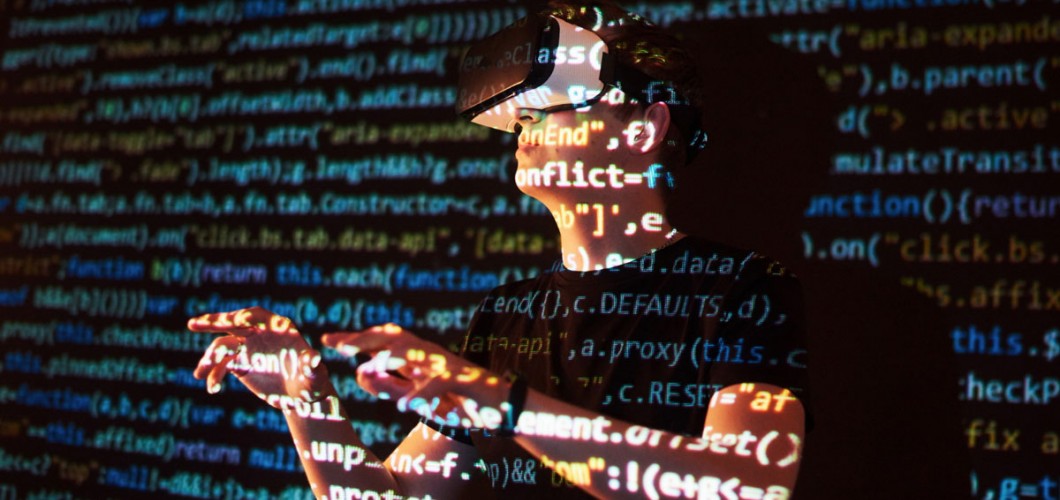
A Comprehensive Guide to Developing Your First Web Application with React
Introduction
React is one of the most popular JavaScript libraries for modern web development. Developed and maintained by Facebook, React simplifies the process of building user interfaces. In this guide, we'll build a simple yet effective web application from scratch using React.
Why Choose React?
- Component-Based Architecture: Break your code into reusable components.
- Virtual DOM: Enhances performance.
- Rich Ecosystem: Supported by numerous open-source libraries.
- Easy to Learn: Quick to grasp with basic JavaScript knowledge.
Setting Up the Development Environment
1. Install Node.js and npm
You need Node.js and npm installed on your system for React projects.
node -v
npm -v
If not installed, download them from the official Node.js website.
2. Start a Project with create-react-app
create-react-app
is a CLI tool that helps you start React projects quickly.
npx create-react-app my-first-react-app
cd my-first-react-app
npm start
This will start a local server and launch a React project.
Understanding the Project Structure
my-first-react-app/
├── node_modules/
├── public/
│ └── index.html
├── src/
│ ├── App.js
│ ├── App.css
│ └── index.js
├── package.json
└── README.md
- public/index.html: The main HTML file.
- src/index.js: The entry point of the React application.
- src/App.js: The main application component.
Creating Your First React Component
Edit the App.js File
import React from 'react';
function App() {
return (
<div className="App">
<h1>Hello, React!</h1>
<p>Welcome to your first React application.</p>
</div>
);
}
export default App;
Start Your Application
npm start
Visit http://localhost:3000
in your browser.
Working with Components
React components are categorized into Functional and Class-Based.
Functional Component
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
Class-Based Component
import React, { Component } from 'react';
class Welcome extends Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
Managing State with useState
React provides the useState
hook for state management.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default Counter;
Handling Side Effects with useEffect
Use useEffect
for API calls or DOM manipulations.
import React, { useState, useEffect } from 'react';
function UserList() {
const [users, setUsers] = useState([]);
useEffect(() => {
fetch('https://jsonplaceholder.typicode.com/users')
.then(response => response.json())
.then(data => setUsers(data));
}, []);
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
export default UserList;
Routing with React Router
npm install react-router-dom
import { BrowserRouter as Router, Routes, Route, Link } from 'react-router-dom';
function Home() {
return <h2>Home</h2>;
}
function About() {
return <h2>About</h2>;
}
function App() {
return (
<Router>
<nav>
<Link to="/">Home</Link> | <Link to="/about">About</Link>
</nav>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
</Routes>
</Router>
);
}
Styling with CSS
Edit the App.css
file:
.App {
text-align: center;
}
button {
padding: 10px;
background-color: #61dafb;
border: none;
cursor: pointer;
}
Deploying Your Project
npm run build
- The
build/
directory contains the production-ready version. - You can deploy it on platforms like Netlify or Vercel.
Conclusion
Building a simple web application with React is straightforward. In this guide, we explored the core concepts and powerful features of React. Now, you can use these basics to develop more complex applications!
???? What's your favorite React feature? Share in the comments!
Leave a Comment