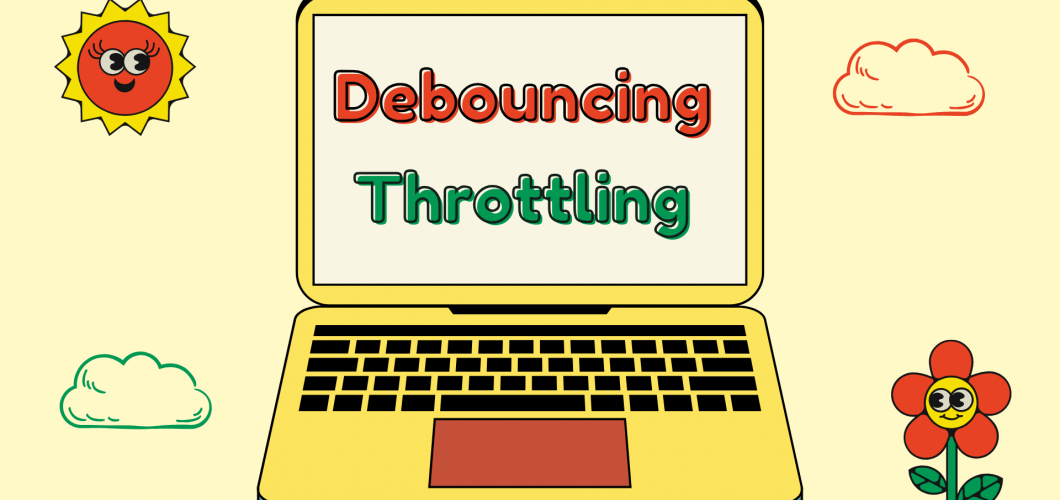
What Are Debouncing and Throttling in JavaScript? A Comprehensive Guide
Introduction
In web development, debouncing and throttling are widely used techniques to enhance user experience and improve performance. These techniques help optimize event listeners by reducing unnecessary function calls, making applications more efficient.
In this article, we will explore debouncing and throttling in detail, explaining how they work and when to use them.
1. What Is Debouncing?
Debouncing is a technique used to prevent a function from executing multiple times within a short period. The function is only triggered after a specified delay when no further event occurs.
How It Works?
A function is delayed until a certain period has passed since the last event occurrence. If the event is triggered again within that period, the timer resets and waits for another interval.
Example Scenario:
When a user types in a search bar, instead of making a request on every keystroke, we can use debouncing to send the request only after the user stops typing for a while.
Debouncing Example:
function debounce(func, delay) {
let timer;
return function(...args) {
clearTimeout(timer);
timer = setTimeout(() => func.apply(this, args), delay);
};
}
function searchQuery(query) {
console.log("API call: " + query);
}
const optimizedSearch = debounce(searchQuery, 500);
document.getElementById("search").addEventListener("input", (event) => {
optimizedSearch(event.target.value);
});
In this code, the debounce
function ensures that searchQuery
is only called after 500ms of no new input.
2. What Is Throttling?
Throttling is a technique that ensures a function is executed only once within a specified time interval, even if an event occurs multiple times.
How It Works?
When an event is triggered multiple times within a short duration, throttling allows the function to execute only once per defined interval and ignores the remaining calls.
Example Scenario:
Scroll events fire continuously, which can cause performance issues. Using throttling, we can limit function execution to occur at most once per second.
Throttling Example:
function throttle(func, limit) {
let inThrottle;
return function(...args) {
if (!inThrottle) {
func.apply(this, args);
inThrottle = true;
setTimeout(() => inThrottle = false, limit);
}
};
}
function logScroll() {
console.log("Page scrolled: " + new Date().toISOString());
}
const optimizedScroll = throttle(logScroll, 1000);
document.addEventListener("scroll", optimizedScroll);
In this example, logScroll
will only execute once per second, even if the scroll event fires multiple times.
3. Comparison of Debouncing and Throttling
Feature | Debouncing | Throttling |
---|---|---|
Purpose | Prevents multiple rapid calls of a function. | Ensures a function executes at most once per interval. |
Use Case | Search box, auto-save functionality. | Scroll, resize, frequent button clicks. |
How It Works | Waits until no event occurs within a delay period. | Executes immediately, then restricts further execution until the interval ends. |
Advantage | Prevents unnecessary API calls. | Enhances performance by limiting function execution. |
Disadvantage | May introduce a delay in function execution. | May not execute the last event if frequent calls stop suddenly. |
4. When to Use Debouncing vs. Throttling?
Situation | Recommended Technique |
---|---|
Sending API requests based on user input | Debouncing |
Loading more content while scrolling | Throttling |
Handling window resize events | Throttling |
Preventing multiple button clicks from triggering duplicate requests | Debouncing |
Waiting for user input completion before triggering an action | Debouncing |
5. Conclusion
Debouncing and throttling are essential techniques for optimizing performance. Debouncing prevents a function from executing multiple times within a short period, while throttling ensures a function runs at most once in a defined interval.
Using these techniques in the right scenarios can significantly enhance your web application's efficiency and responsiveness. ????
Leave a Comment