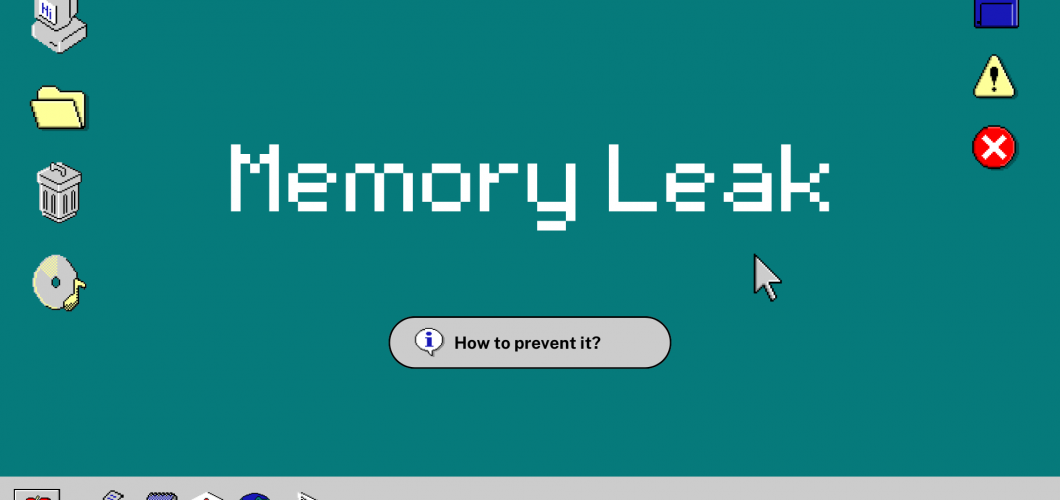
What is Memory Leak in JavaScript and How to Prevent It?
Introduction
In JavaScript, a memory leak occurs when the application continues to use memory unnecessarily. Over time, this leads to increased memory usage and can cause a decrease in performance or even crashes. Memory leaks are often caused by improperly managed variables, objects, or DOM elements. In this post, we will explore what memory leaks in JavaScript are, how they occur, and how to prevent them in detail, with examples and visual aids.
What is a Memory Leak?
When JavaScript runs, memory usage is automatically managed. A memory leak is a situation where a particular object or resource continues to occupy memory unnecessarily over time. This means that even though the system allocated memory for an object, the resource is not released, resulting in the application consuming more memory continuously.
Memory Management and Garbage Collection
JavaScript is an automatically managed memory language, meaning developers do not need to manually release memory. The garbage collector (GC) cleans up unused objects. However, in some cases, this mechanism fails, and memory leaks occur.
Types of Memory Leaks
Global Variables and Objects
Global variables, especially those defined in the global scope, can remain in memory for extended periods. If an object is declared globally, the JavaScript garbage collector won't release it until the application finishes, leading to memory leaks.
var globalObj = { name: 'Memory Leak' }; function createLeak() { globalObj = { name: 'New Object' }; } // When the createLeak function runs, the previous globalObj does not get freed from memory.
Event Listeners and DOM Elements
Event listeners are added to DOM elements to listen for events. If an event listener is added but never removed, it holds the DOM element in memory and prevents it from being garbage collected, causing a memory leak.
const button = document.getElementById('myButton'); button.addEventListener('click', function() { console.log('Button clicked!'); }); // If the event listener is not removed, the 'button' element will remain in memory.
Closures
In JavaScript, a function can be nested within another function. The inner function has access to the outer function's variables. If these variables are leaked when the outer function returns, they can remain in memory, causing a memory leak.
function createClosure() { let largeArray = new Array(1000000).fill(0); return function() { console.log(largeArray[0]); }; } const closure = createClosure(); // largeArray remains in memory because it's referenced by the closure function.
setTimeout and setInterval Usage
setTimeout
andsetInterval
are used to schedule functions to be executed after a specified time or at regular intervals. If these timers are not cleared properly (e.g., usingclearTimeout
orclearInterval
), they can lead to memory leaks.let timer = setInterval(() => { console.log('Timer running...'); }, 1000); // If clearInterval is not used, the timer will not be freed from memory.
How to Prevent Memory Leaks?
1. Avoid Global Variables
Global variables, particularly those declared in the global scope, can lead to memory leaks. Instead, use local variables whenever possible.
// Global Variable: Avoid it!
var globalObj = { name: 'Memory Leak' };
// Local Variable: Recommended
function processData() {
let localObj = { name: 'No Leak' };
}
2. Clean Up Event Listeners
Event listeners that are added to DOM elements can prevent these elements from being freed if not properly removed. Always remove event listeners when they are no longer needed.
const button = document.getElementById('myButton');
function handleClick() {
console.log('Button clicked!');
}
button.addEventListener('click', handleClick);
// Removing the event listener
button.removeEventListener('click', handleClick);
3. Clear setTimeout and setInterval
setTimeout
and setInterval
timers can cause memory leaks if they are not properly cleared. Always clear them using clearTimeout
or clearInterval
after they are no longer needed.
let timer = setInterval(() => {
console.log('Running...');
}, 1000);
// Clear the interval
clearInterval(timer);
4. Use Weak References
Data structures like WeakMap
or WeakSet
can help prevent memory leaks. These structures hold objects with weak references, which means the objects are automatically removed from memory by garbage collection if there are no strong references to them.
let weakMap = new WeakMap();
let obj = {};
weakMap.set(obj, 'some value');
obj = null; // Since there are no other references, the WeakMap entry is cleaned up.
5. Use Closures Wisely
Closures allow inner functions to access variables from outer functions. However, if closures unnecessarily retain large data sets, they can lead to memory leaks. Avoid large data references in closures.
function createClosure() {
let largeData = new Array(1000000).fill(0);
return function() {
console.log(largeData[0]);
};
}
// In this case, largeData will remain in memory because of the closure.
Conclusion
Memory leaks in JavaScript can lead to serious performance issues in software development. Therefore, careful attention to memory management is essential, and the techniques mentioned above should be applied to minimize memory leaks. While garbage collection handles most of the work automatically, developers need to manually monitor and control memory usage in certain scenarios. By preventing memory leaks, you can develop faster, more efficient, and user-friendly applications.
Leave a Comment